Below, you’ll find a detailed guide on how to add the Auto iFrame Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Auto iFrame Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the Auto iFrame Plugin and the shortcodes it provides:
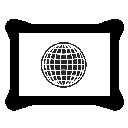
"Auto iFrame is a WordPress plugin that simplifies the process of embedding iFrames into your posts or pages. It offers a user-friendly interface and seamless integration."
- [auto-iframe]
Auto iFrame [auto-iframe] Shortcode
The Auto-iFrame shortcode is a versatile tool that allows embedding of iFrames into WordPress posts or pages. It simplifies the process of adding iFrames, providing a range of customizable attributes such as link, tag, width, height, autosize, fudge, border, scroll, and query.
Shortcode: [auto-iframe]
Parameters
Here is a list of all possible auto-iframe shortcode parameters and attributes:
link
– Required. The URL of the source for the iFrame.tag
– Optional. Unique identifier for multiple iFrames on a page.width
– Optional. Specifies the width of the iFrame.height
– Optional. Specifies the initial height of the iFrame.autosize
– Optional. Enables auto resizing of the iFrame based on content.fudge
– Optional. Fudge factor to apply when changing the height.border
– Optional. Enables the border on the iFrame.scroll
– Optional. Enables the scroll bar on the iFrame.query
– Optional. Passes the parent’s page query string to the iFrame.
Examples and Usage
Basic example – Embeds an iFrame with a specified link.
[auto-iframe link="https://www.example.com" /]
Advanced examples
Embeds an iFrame with a specified link, width, and height. Autosizing is disabled and a unique tag is used for identification.
[auto-iframe link="https://www.example.com" tag="myiframe" width="500px" height="400px" autosize="no" /]
Embeds an iFrame with a specified link and enables autosizing. A unique tag is used for identification, a border is added, and the scroll bar is enabled.
[auto-iframe link="https://www.example.com" tag="myiframe2" autosize="yes" border="1" scroll="yes" /]
Embeds an iFrame with a specified link, width, height, and fudge factor. Autosizing is enabled, a unique tag is used for identification, and the parent’s page query string is passed to the iFrame.
[auto-iframe link="https://www.example.com" tag="myiframe3" width="100%" height="200px" autosize="yes" fudge="100" query="yes" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [auto-iframe]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'auto-iframe', 'auto_iframe_shortcode' );
Shortcode PHP function:
function auto_iframe_shortcode( $atts ) {
/*
Auto iFrame shortcode is in the format of:
[auto-iframe link=xxx tag=xxx width=xxx height=xxx autosize=yes/no]
Where:
link = the url of the source for the iFrame. REQUIRED.
tag = a unique identifier in case you want more than one iFrame on a page. Default = auto-iframe.
width = width of the iFrame (100% by default). Can be % or px. Default = 100%.
height = the initial height of the iframe (100% by default). Can be % or px. Default = 100%.
autosize = enable the auto sizing of the iFrame based on the content. The initial height of the iFrame will be set to "height" and then resized. Default = true.
fudge = a fudge factor to apply when changing the height (integer number, no "px"). Default = 50.
border = enable the border on the iFrame. Default = 0.
scroll = enable the scroll bar on the iFrame. Default = no.
query = pass the parent's page query string to the iFrame. Default = no.
*/
// We don't have any parameters, just return a blank string.
if( !is_array( $atts ) ) { return ''; }
// Get the link.
$link = '';
if( array_key_exists( 'link', $atts ) ) { $link = htmlentities(trim( $atts['link'] ), ENT_QUOTES ); }
// If no link has been passed in, there's nothing to do so just return a blank string.
if( $link == '' ) { return ''; }
// Get the rest of the attributes.
$tag = 'auto-iframe';
if( array_key_exists( 'tag', $atts ) ) { $tag = htmlentities(trim( $atts['tag'] ), ENT_QUOTES ); }
$width = '100%';
if( array_key_exists( 'width', $atts ) ) { $width = htmlentities(trim( $atts['width'] ), ENT_QUOTES ); }
$height = 'auto';
if( array_key_exists( 'height', $atts ) ) { $height = htmlentities(trim( $atts['height'] ), ENT_QUOTES ); }
$autosize = true;
if( array_key_exists( 'autosize', $atts ) ) { if( strtolower( $atts['autosize'] ) != 'yes' ) { $autosize = false; } ; }
$fudge = 50;
if( array_key_exists( 'fudge', $atts ) ) { $fudge = intval( $atts['fudge'] ); }
$border = '0';
if( array_key_exists( 'border', $atts ) ) { $border = htmlentities(trim( $atts['border'] ), ENT_QUOTES ); }
$scroll = 'no';
if( array_key_exists( 'scroll', $atts ) ) { if( array_key_exists( 'autosize', $atts ) && strtolower( $atts['autosize'] ) != 'yes' ) { $scroll = 'yes'; } ; }
if( array_key_exists( 'query', $atts ) ) {
$qs_len = strlen( $_SERVER['QUERY_STRING'] );
if( strstr( $link, '?' ) === FALSE && $qs_len > 0 ) {
$link = $link . '?' . $_SERVER['QUERY_STRING'];
} else if( $qs_len > 0 ) {
$link = $link . '&' . $_SERVER['QUERY_STRING'];
}
}
$onload_autosize = '';
$result = '';
if( $autosize ) {
// Enqueue the javascript and jquery code.
wp_enqueue_script( 'auto_iframe_js', plugins_url( 'auto-iframe.js', __FILE__ ), array( 'jquery' ) );
$result = '<script type="text/javascript">// <![CDATA[' . "\n";
$result .= 'jQuery(document).ready(function(){' . "\n";
$result .= ' setInterval( function() { AutoiFrameAdjustiFrameHeight( \'' . $tag . '\', ' . $fudge .'); }, 1000 );' . "\n";
$result .= '});' . "\n";
$result .= '// ]]></script>' . "\n";
$onload_autosize = 'onload="AutoiFrameAdjustiFrameHeight(\'' . $tag . '\',' . $fudge . ');"';
}
$result .= '<iframe id="' . $tag . '" name="' . $tag . '" src="' . $link . '" width="' . $width . '" height="' . $height . '" frameborder="' . $border . '" scrolling="' . $scroll . '"' . $onload_autosize . '></iframe>';
return $result;
}
Code file location:
auto-iframe/auto-iframe/auto-iframe.php
Conclusion
Now that you’ve learned how to embed the Auto iFrame Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply