Below, you’ll find a detailed guide on how to add the WP-FormAssembly Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the WP-FormAssembly Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the WP-FormAssembly Plugin and the shortcodes it provides:
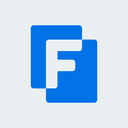
"WP-FormAssembly is a versatile WordPress plugin that simplifies the creation and management of web forms. Easy to use, it enhances user experience and streamlines data collection."
- [formassembly]
WP-FormAssembly [formassembly] Shortcode
The FormAssembly shortcode is designed to embed FormAssembly forms into WordPress posts or pages. It uses either the iframe or REST API method. The shortcode takes attributes like ‘server’, ‘formid’, ‘workflowid’, ‘style’, and ‘iframe’. Depending on the attributes, it generates an iframe or makes a REST API call to fetch the form. It also includes error handling for invalid URLs and unsupported publishing methods.
Shortcode: [formassembly]
Parameters
Here is a list of all possible formassembly shortcode parameters and attributes:
server
– URL of the FormAssembly serverformid
– ID of the form to be displayedworkflowid
– ID of the workflow to be startedstyle
– CSS styling to be applied to the formiframe
– If set, form is displayed in an iframe
Examples and Usage
Basic example – Display a form using the form’s ID:
[formassembly formid=123 /]
Here, the shortcode is used to display the form with the ID 123. The form will be fetched from the default server “https://app.formassembly.com”.
Advanced examples
Display a form using the form’s ID, with a specified server:
[formassembly formid=123 server="https://yourserver.com" /]
In this example, the shortcode is used to display the form with the ID 123. However, instead of fetching the form from the default server, it fetches the form from the specified server “https://yourserver.com”.
Display a form using a workflow ID, with a specified server and style:
[formassembly workflowid=456 server="https://yourserver.com" style="width: 80%; margin: 0 auto;" /]
This example demonstrates the use of the shortcode to display a form using a workflow ID. The form is fetched from the specified server and a custom style is applied to the form.
Display a form in an iframe:
[formassembly formid=123 iframe="1" /]
This example shows how to display a form in an iframe. The form with the ID 123 is fetched from the default server and displayed in an iframe.
PHP Function Code
In case you have difficulties debugging what causing issues with [formassembly]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('formassembly', 'fa_add');
Shortcode PHP function:
function fa_add($atts)
{
$qs = isset($_SERVER['QUERY_STRING']) ? wp_sanitize_redirect($_SERVER['QUERY_STRING']) : '';
if (!empty($qs)) {
$qs = '?' . $qs;
};
if (isset($atts['server'])) {
if (wp_http_validate_url($atts['server']) === false) {
return '';
}
$host_url = $atts['server'];
} else {
$host_url = "https://app.formassembly.com";
}
$new_content = '';
if (isset($atts['formid']) || isset($atts['workflowid'])) {
$action_url = "forms/view";
$fa_id = $atts['formid'];
if (isset($atts['workflowid'])) {
$action_url = "workflows/start";
$fa_id = $atts['workflowid'];
}
// Add style options in to combat wordpresses' default centering of forms.
if (!isset($atts['style'])) {
$style = "<style>.wForm form{text-align: left;}</style>";
} else {
$style = "<style>.wForm form{" . $atts['style'] . "}</style>";
}
if (isset($atts['iframe'])) {
// IFRAME method
/**
* Add jsid to maintain session in browsers that block cookies.
* Setting as null informs the server we are in an iframe without
* an active session.
*/
$qs .= (strpos($qs, '?') !== false ? '&' : '?') . 'jsid=';
$url = $host_url . '/' . $action_url . '/' . $fa_id . $qs;
// validate url
if (!wp_http_validate_url($url)) {
return $style . "<div style=\"color:red;margin-left:auto;margin-right:auto;\">Invalid url added to server attribute to your FormAssembly tag.</div>";
}
if (!isset($atts['style'])) {
$atts['style'] = "width: 100%; min-height: 650px;";
}
$attributes = implode(' ', array("frameborder=0", "style='" . $atts['style'] . "'"));
$new_content = '<iframe ' . $attributes . ' src="' . $url . '"></iframe>';
} else {
// REST API method
if (
isset($_GET['tfa_next']) &&
isset($atts['formid']) &&
isTfaNextInvalid($_GET['tfa_next'], $fa_id)
) {
return $style . "<div style=\"color:red;margin-left:auto;margin-right:auto;\">Invalid url provided in tfa_next parameter</div>";
}
if (
isset($_GET['tfa_next']) &&
isset($atts['workflowid']) &&
isTfaNextInvalidForWorkflowId($_GET['tfa_next'], $fa_id)
) {
return $style . "<div style=\"color:red;margin-left:auto;margin-right:auto;\">Invalid url provided in tfa_next parameter</div>";
}
if (!isset($_GET['tfa_next'])) {
$url = $host_url . '/rest/' . $action_url . '/' . $fa_id . $qs;
} else {
$url = $host_url . '/rest' . wp_sanitize_redirect($_GET['tfa_next']);
}
//validate url
if (!wp_http_validate_url($url)) {
return $style . "<div style=\"color:red;margin-left:auto;margin-right:auto;\">Invalid url added to server attribute to your FormAssembly tag.</div>";
}
if (function_exists("wp_remote_get")) {
$response = wp_remote_get($url);
$responseCode = wp_remote_retrieve_response_code($response);
$responseBody = wp_remote_retrieve_body($response);
if ($responseCode != 200) {
return $style . "<div style=\"color:red;margin-left:auto;margin-right:auto;\">" . $responseCode . '<br>' . $responseBody . "</div>";
}
$buffer = $responseBody;
} else {
// REST API call not supported, must use iframe instead.
$buffer = "<div style=\"color:red;margin-left:auto;margin-right:auto;\">Your server does not support this form publishing method. Try adding iframe=\"1\" to your FormAssembly tag.</div>";
}
$new_content = $style . $buffer;
}
}
return $new_content;
}
Code file location:
formassembly-web-forms/formassembly-web-forms/wp_formassembly.php
Conclusion
Now that you’ve learned how to embed the WP-FormAssembly Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply