Below, you’ll find a detailed guide on how to add the Instagram Feed Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Instagram Feed Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the Instagram Feed Plugin and the shortcodes it provides:
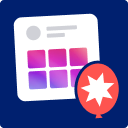
"Smash Balloon Social Photo Feed is the best social feed plugin for WordPress. This plugin, also known as 'instagram-feed', effortlessly integrates Instagram feeds into your WordPress site."
- [instagram-feed]
Instagram Feed [instagram-feed] Shortcode
The Instagram-Feed shortcode is used to display Instagram feeds on your WordPress site. It fetches data from Instagram, checks for errors, and if none, it displays the feed. The PHP code associated with the shortcode ensures proper data retrieval, error handling, and feed display. It also manages cache for improved performance and checks user capabilities for error visibility. In case of errors, it generates a user-friendly message. It also handles image resizing and JavaScript image loading based on the settings. The feed display is customizable with given attributes.
Shortcode: [instagram-feed]
Examples and Usage
Basic example – Displaying Instagram feed using the shortcode without any additional parameters. This will display the Instagram feed according to the default settings in your Instagram Feed plugin.
[instagram-feed]
Advanced examples
Displaying an Instagram feed with a specific number of photos. This shortcode takes an additional parameter ‘num’ which specifies the number of photos to display from the feed.
[instagram-feed num=10]
Displaying an Instagram feed from a specific user. This shortcode takes an additional parameter ‘username’ which specifies the Instagram username for the feed to display.
[instagram-feed username="exampleuser"]
Displaying an Instagram feed with a specific number of columns. This shortcode takes an additional parameter ‘cols’ which specifies the number of columns to display the feed in.
[instagram-feed cols=3]
Combining multiple parameters. This shortcode combines the ‘num’, ‘username’, and ‘cols’ parameters to display a specific number of photos from a specific user in a specific number of columns.
[instagram-feed num=10 username="exampleuser" cols=3]
PHP Function Code
In case you have difficulties debugging what causing issues with [instagram-feed]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('instagram-feed', 'display_instagram');
Shortcode PHP function:
function display_instagram( $atts = array(), $preview_settings = false ) {
do_action( 'sbi_before_display_instagram' );
$database_settings = sbi_get_database_settings();
if ( $database_settings['sb_instagram_ajax_theme'] !== 'on'
&& $database_settings['sb_instagram_ajax_theme'] !== 'true'
&& $database_settings['sb_instagram_ajax_theme'] !== '1'
&& $database_settings['sb_instagram_ajax_theme'] !== true ) {
wp_enqueue_script( 'sbi_scripts' );
}
if ( $database_settings['enqueue_css_in_shortcode'] === 'on' || $database_settings['enqueue_css_in_shortcode'] === 'true' || $database_settings['enqueue_css_in_shortcode'] === true ) {
wp_enqueue_style( 'sbi_styles' );
}
$instagram_feed_settings = new SB_Instagram_Settings( $atts, $database_settings, $preview_settings );
$early_settings = $instagram_feed_settings->get_settings();;
if ( empty( $early_settings ) && ! sbi_doing_customizer( $atts ) ) {
$style = current_user_can( 'manage_instagram_feed_options' ) ? ' style="display: block;"' : '';
$id = isset( $atts['feed'] ) ? (int)$atts['feed'] : false;
if ( $id ) {
$message = sprintf( __( 'Error: No feed with the ID %s found.', 'instagram-feed' ), $id );
} else {
$message = __( 'Error: No feed found.', 'instagram-feed' );
}
ob_start(); ?>
<div id="sbi_mod_error" <?php echo $style; ?>>
<span><?php esc_html_e('This error message is only visible to WordPress admins', 'instagram-feed' ); ?></span><br />
<p><strong><?php echo esc_html( $message ); ?></strong>
<p><?php esc_html_e( 'Please go to the Instagram Feed settings page to create a feed.', 'instagram-feed' ); ?></p>
</div>
<?php
$html = ob_get_contents();
ob_get_clean();
return $html;
}
$instagram_feed_settings->set_feed_type_and_terms();
$instagram_feed_settings->set_transient_name();
$transient_name = $instagram_feed_settings->get_transient_name();
$settings = $instagram_feed_settings->get_settings();
$feed_type_and_terms = $instagram_feed_settings->get_feed_type_and_terms();
$instagram_feed = new SB_Instagram_Feed( $transient_name );
$instagram_feed->set_cache( $instagram_feed_settings->get_cache_time_in_seconds(), $settings );
if ( $settings['caching_type'] === 'background' ) {
$instagram_feed->add_report( 'background caching used' );
if ( $instagram_feed->regular_cache_exists() ) {
$instagram_feed->add_report( 'setting posts from cache' );
$instagram_feed->set_post_data_from_cache();
}
if ( $instagram_feed->need_to_start_cron_job() ) {
$instagram_feed->add_report( 'setting up feed for cron cache' );
$to_cache = array(
'atts' => $atts,
'last_requested' => time(),
);
$instagram_feed->set_cron_cache( $to_cache, $instagram_feed_settings->get_cache_time_in_seconds() );
SB_Instagram_Cron_Updater::do_single_feed_cron_update( $instagram_feed_settings, $to_cache, $atts, false );
$instagram_feed->set_cache( $instagram_feed_settings->get_cache_time_in_seconds(), $settings );
$instagram_feed->set_post_data_from_cache();
} elseif ( $instagram_feed->should_update_last_requested() ) {
$instagram_feed->add_report( 'updating last requested' );
$to_cache = array(
'last_requested' => time(),
);
$instagram_feed->set_cron_cache( $to_cache, $instagram_feed_settings->get_cache_time_in_seconds(), $settings['backup_cache_enabled'] );
}
} elseif ( $instagram_feed->regular_cache_exists() ) {
$instagram_feed->add_report( 'page load caching used and regular cache exists' );
$instagram_feed->set_post_data_from_cache();
if ( $instagram_feed->need_posts( $settings['num'] ) && $instagram_feed->can_get_more_posts() ) {
while ( $instagram_feed->need_posts( $settings['num'] ) && $instagram_feed->can_get_more_posts() ) {
$instagram_feed->add_remote_posts( $settings, $feed_type_and_terms, $instagram_feed_settings->get_connected_accounts_in_feed() );
}
$instagram_feed->cache_feed_data( $instagram_feed_settings->get_cache_time_in_seconds(), $settings['backup_cache_enabled'] );
}
} else {
$instagram_feed->add_report( 'no feed cache found' );
while ( $instagram_feed->need_posts( $settings['num'] ) && $instagram_feed->can_get_more_posts() ) {
$instagram_feed->add_remote_posts( $settings, $feed_type_and_terms, $instagram_feed_settings->get_connected_accounts_in_feed() );
}
if ( ! $instagram_feed->should_use_backup() ) {
$instagram_feed->cache_feed_data( $instagram_feed_settings->get_cache_time_in_seconds(), $settings['backup_cache_enabled'] );
}
}
if ( $instagram_feed->should_use_backup() ) {
$instagram_feed->add_report( 'trying to use backup' );
$instagram_feed->maybe_set_post_data_from_backup();
$instagram_feed->maybe_set_header_data_from_backup();
}
// if need a header
if ( $instagram_feed->need_header( $settings, $feed_type_and_terms ) ) {
if ( $instagram_feed->should_use_backup() && $settings['minnum'] > 0 ) {
$instagram_feed->add_report( 'trying to set header from backup' );
$header_cache_success = $instagram_feed->maybe_set_header_data_from_backup();
} elseif ( $instagram_feed->regular_header_cache_exists() ) {
// set_post_data_from_cache
$instagram_feed->add_report( 'page load caching used and regular header cache exists' );
$instagram_feed->set_header_data_from_cache();
} else {
$instagram_feed->add_report( 'no header cache exists' );
$instagram_feed->set_remote_header_data( $settings, $feed_type_and_terms, $instagram_feed_settings->get_connected_accounts_in_feed() );
$instagram_feed->cache_header_data( $instagram_feed_settings->get_cache_time_in_seconds(), $settings['backup_cache_enabled'] );
}
} else {
$instagram_feed->add_report( 'no header needed' );
}
if ( $settings['resizeprocess'] === 'page' ) {
$instagram_feed->add_report( 'resizing images for post set' );
$post_data = $instagram_feed->get_post_data();
$post_data = array_slice( $post_data, 0, $settings['num'] );
$post_set = new SB_Instagram_Post_Set( $post_data, $transient_name );
$post_set->maybe_save_update_and_resize_images_for_posts();
}
if ( $settings['disable_js_image_loading'] || $settings['imageres'] !== 'auto' ) {
global $sb_instagram_posts_manager;
$post_data = $instagram_feed->get_post_data();
if ( ! $sb_instagram_posts_manager->image_resizing_disabled() ) {
$image_ids = array();
foreach ( $post_data as $post ) {
$image_ids[] = SB_Instagram_Parse::get_post_id( $post );
}
$resized_images = SB_Instagram_Feed::get_resized_images_source_set( $image_ids, 0, $transient_name );
$instagram_feed->set_resized_images( $resized_images );
}
}
return $instagram_feed->get_the_feed_html( $settings, $atts, $instagram_feed_settings->get_feed_type_and_terms(), $instagram_feed_settings->get_connected_accounts_in_feed() );
}
Code file location:
instagram-feed/instagram-feed/inc/if-functions.php
Conclusion
Now that you’ve learned how to embed the Instagram Feed Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply