Below, you’ll find a detailed guide on how to add the Order Tracking Shortcodes to your WordPress website, including their parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Order Tracking Plugin shortcodes not to show or not to work correctly.
Before starting, here is an overview of the Order Tracking Plugin and the shortcodes it provides:
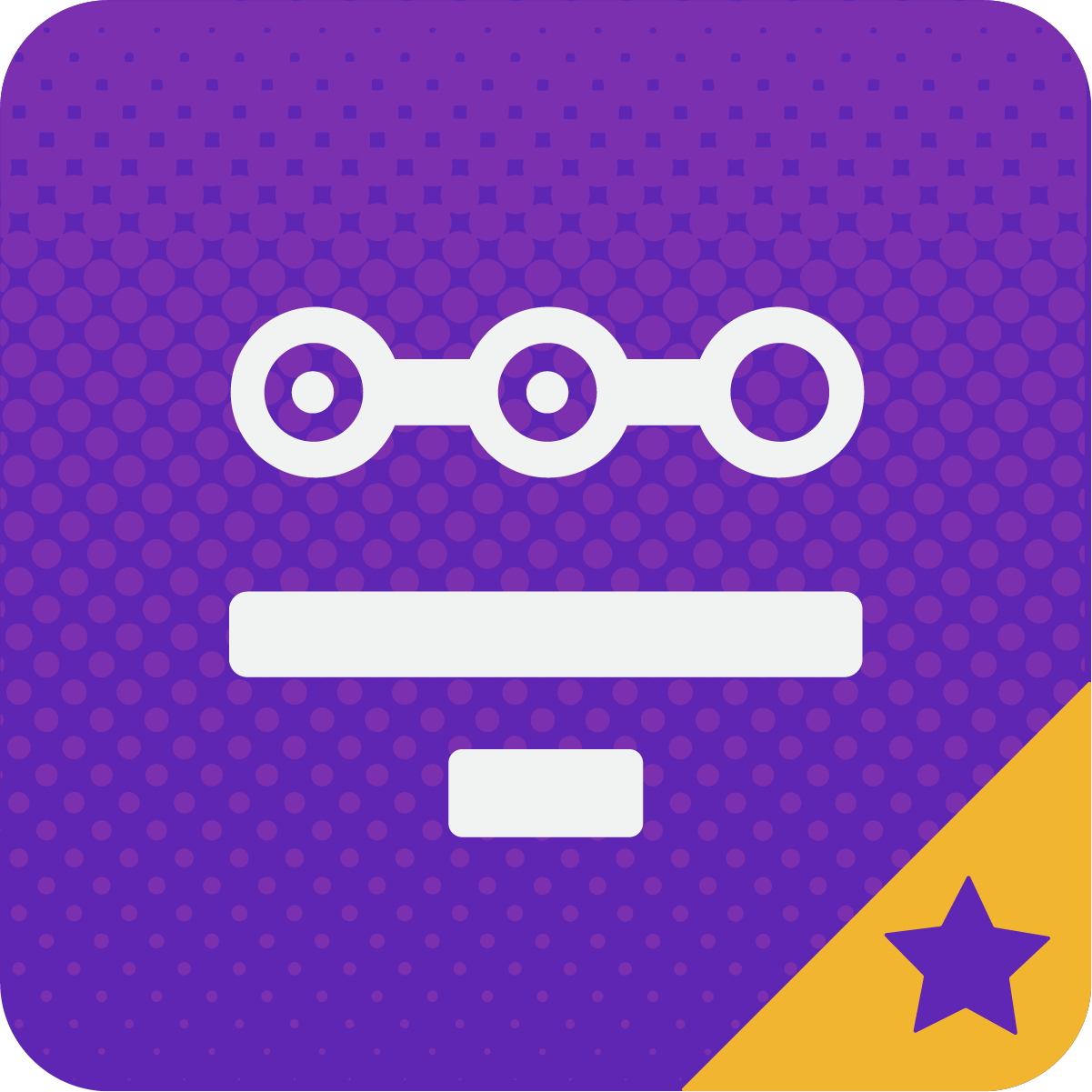
"Order Tracking – WordPress Status Tracking Plugin is a dynamic tool for keeping track of orders or projects. Its user-friendly interface and advanced features ensure seamless management of tasks."
- [tracking-form]
- [customer-form]
- [sales-rep-form]
- [customer-order]
- [order-number-search]
Order Tracking [tracking-form] Shortcode
The EWD Order Tracking shortcode allows users to track their orders on the front-end. It generates a form where users can input their order details. The shortcode attributes can be customized to show orders, set form title, order field text, email field text, order instructions, submit text, and notes submit. It also has a filter to modify the accepted attributes and an update feature to change order status and location.
Shortcode: [tracking-form]
Parameters
Here is a list of all possible tracking-form shortcode parameters and attributes:
show_orders
– determines if the order history is displayed or notorder_form_title
– sets the title of the order tracking formorder_field_text
– changes the placeholder text of the order fieldemail_field_text
– changes the placeholder text of the email fieldorder_instructions
– allows for additional instructions to be added to the formsubmit_text
– changes the text on the submission buttonnotes_submit
– provides a field for additional notes on the form
Examples and Usage
Basic example – Displays a tracking form without any customization.
[tracking-form]
Advanced examples
Customizes the tracking form with a title, order field text, and email field text. The form will display the title “Track Your Order”, order field text as “Order Number”, and email field text as “Your Email”.
[tracking-form order_form_title="Track Your Order" order_field_text="Order Number" email_field_text="Your Email"]
Displays a tracking form with instructions and custom submit button text. The form will display the instructions “Please enter your order details below” and the submit button will be labeled “Track”.
[tracking-form order_instructions="Please enter your order details below" submit_text="Track"]
Displays a tracking form with a custom note submit button text. The form will display the note submit button text as “Submit Notes”.
[tracking-form notes_submit="Submit Notes"]
Displays a tracking form with all available customization options.
[tracking-form show_orders="yes" order_form_title="Track Your Order" order_field_text="Order Number" email_field_text="Your Email" order_instructions="Please enter your order details below" submit_text="Track" notes_submit="Submit Notes"]
PHP Function Code
In case you have difficulties debugging what causing issues with [tracking-form]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'tracking-form', 'ewd_otp_tracking_form_shortcode' );
Shortcode PHP function:
function ewd_otp_tracking_form_shortcode( $atts ) {
global $ewd_otp_controller;
// Define shortcode attributes
$order_atts = array(
'show_orders' => 'no',
'order_form_title' => '',
'order_field_text' => '',
'email_field_text' => '',
'order_instructions' => '',
'submit_text' => '',
'notes_submit' => '',
);
// Create filter so addons can modify the accepted attributes
$order_atts = apply_filters( 'ewd_otp_tracking_form_shortcode_atts', $order_atts );
// Extract the shortcode attributes
$args = shortcode_atts( $order_atts, $atts );
// Render booking form
ewd_otp_load_view_files();
// Possibly update order status and location from the front-end
$update = ewd_otp_possibly_update_order();
if ( $update ) {
$args['update_message'] = $update;
}
$order_form = new ewdotpViewOrderForm( $args );
$order_form->set_request_parameters();
$output = $order_form->render();
return $output;
}
Code file location:
order-tracking/order-tracking/includes/template-functions.php
Order Tracking [customer-form] Shortcode
The ‘customer-form’ shortcode is used in the Order Tracking Plugin to create a customer form. It verifies the customer’s email, fetches customer details, and allows order downloads.
Shortcode: [customer-form]
Examples and Usage
Basic example – Displays a customer form using the default settings.
[customer-form /]
PHP Function Code
In case you have difficulties debugging what causing issues with [customer-form]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'customer-form', 'ewd_otp_customer_form_shortcode' );
Shortcode PHP function:
function ewd_otp_customer_form_shortcode( $atts ) {
global $ewd_otp_controller;
// Define shortcode attributes
$customer_atts = array(
'order_form_title' => '',
'order_field_text' => '',
'email_field_text' => '',
'order_instructions' => '',
'submit_text' => '',
);
$verify_email = $ewd_otp_controller->settings->get_setting( 'email-verification' );
if ( ! empty( $_POST['ewd_otp_identifier_number'] ) and ( ! empty( $_POST['ewd_otp_form_type'] ) and $_POST['ewd_otp_form_type'] == 'customer_form' ) ) {
$customer_id = intval( $_POST['ewd_otp_identifier_number'] );
}
elseif ( get_current_user_id() ) {
$customer_id = $ewd_otp_controller->customer_manager->get_customer_id_from_wp_id( get_current_user_id() );
$verify_email = false;
}
elseif ( class_exists( 'FEUP_User' ) ) {
$feup_user = new FEUP_User();
$customer_id = $ewd_otp_controller->customer_manager->get_customer_id_from_feup_id( $feup_user->Get_User_ID() );
$verify_email = false;
}
if ( ! empty( $customer_id ) ) {
$customer = new ewdotpCustomer();
$customer->load_customer_from_id( $customer_id );
if ( ! $verify_email or $customer->verify_customer_email( sanitize_email( $_POST['ewd_otp_form_email'] ) ) ) {
$customer_atts['customer'] = $customer;
}
}
if ( ! empty( $_POST['ewd_otp_customer_download'] ) and $ewd_otp_controller->settings->get_setting( 'allow-customer-downloads' ) ) {
$customer_id = intval( $_POST['ewd_otp_customer_id'] );
$customer_email = sanitize_email( $_POST['ewd_otp_customer_email'] );
$customer = new ewdotpCustomer();
$customer->load_customer_from_id( $customer_id );
if ( $customer->verify_customer_email( $customer_email ) ) {
$ewd_otp_controller->exports->nonce_check = false;
$ewd_otp_controller->exports->customer_id = $customer_id;
$ewd_otp_controller->exports->after = date( 'Y-m-d H:i:s', strtotime( '-365 days' ) );
$ewd_otp_controller->exports->export_orders();
}
}
// Create filter so addons can modify the accepted attributes
$customer_atts = apply_filters( 'ewd_otp_customer_form_shortcode_atts', $customer_atts );
// Extract the shortcode attributes
$args = shortcode_atts( $customer_atts, $atts );
// Render booking form
ewd_otp_load_view_files();
// Possibly update order status and location from the front-end
$update = ewd_otp_possibly_update_order();
if ( $update ) {
$args['update_message'] = $update;
}
$customer_form = new ewdotpViewCustomerForm( $args );
$customer_form->set_request_parameters();
$output = $customer_form->render();
return $output;
}
Code file location:
order-tracking/order-tracking/includes/template-functions.php
Order Tracking [sales-rep-form] Shortcode
The ‘sales-rep-form’ shortcode from the Order Tracking Plugin allows sales representatives to track their orders. It verifies the user’s permission, fetches the sales rep’s details, and validates the entered email. If an order is updated, it displays a message. The shortcode also allows for download of sales rep orders if enabled in settings. The final output is a rendered sales rep order form.
Shortcode: [sales-rep-form]
Parameters
Here is a list of all possible sales-rep-form shortcode parameters and attributes:
order_form_title
– Specifies the title of the sales rep order form.order_field_text
– Defines the text for the order field.email_field_text
– Sets the text for the email field.order_instructions
– Provides instructions for the order form.submit_text
– Determines the text on the form’s submit button.
Examples and Usage
Basic example – A simple usage of the shortcode without any parameters. This will display the sales representative form with default settings.
[sales-rep-form /]
Advanced examples
The shortcode allows for customization of the form through various parameters. Here are some examples:
Display the sales representative form with a custom title and instructions.
[sales-rep-form order_form_title="Custom Title" order_instructions="Please fill in the form accurately." /]
Customize the text for the order and email fields, and also the text of the submit button.
[sales-rep-form order_field_text="Order Number" email_field_text="Your Email" submit_text="Submit Form" /]
Combine multiple parameters to fully customize the form.
[sales-rep-form order_form_title="Custom Title" order_field_text="Order Number" email_field_text="Your Email" order_instructions="Please fill in the form accurately." submit_text="Submit Form" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [sales-rep-form]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'sales-rep-form', 'ewd_otp_sales_rep_form_shortcode' );
Shortcode PHP function:
function ewd_otp_sales_rep_form_shortcode( $atts ) {
global $ewd_otp_controller;
if ( ! $ewd_otp_controller->permissions->check_permission( 'sales_reps' ) ) { return; }
// Define shortcode attributes
$sales_rep_atts = array(
'order_form_title' => '',
'order_field_text' => '',
'email_field_text' => '',
'order_instructions' => '',
'submit_text' => '',
);
if ( get_current_user_id() ) {
$sales_rep_id = $ewd_otp_controller->sales_rep_manager->get_sales_rep_id_from_wp_id( get_current_user_id() );
}
if ( ! empty( $sales_rep_id ) ) {
$sales_rep = new ewdotpSalesRep();
$sales_rep->load_sales_rep_from_id( $sales_rep_id );
$sales_rep_atts['sales_rep'] = $sales_rep;
}
if ( ! empty( $_POST['ewd_otp_sales_rep_download'] ) and $ewd_otp_controller->settings->get_setting( 'allow-sales-rep-downloads' ) ) {
$sales_rep_id = intval( $_POST['ewd_otp_sales_rep_id'] );
$sales_rep_email = sanitize_email( $_POST['ewd_otp_sales_rep_email'] );
$sales_rep = new ewdotpSalesRep();
$sales_rep->load_sales_rep_from_id( $sales_rep_id );
if ( $sales_rep->verify_sales_rep_email( $sales_rep_email ) ) {
$ewd_otp_controller->exports->nonce_check = false;
$ewd_otp_controller->exports->sales_rep_id = $sales_rep_id;
$ewd_otp_controller->exports->after = date( 'Y-m-d H:i:s', strtotime( '-365 days' ) );
$ewd_otp_controller->exports->export_orders();
}
}
// Create filter so addons can modify the accepted attributes
$sales_rep_atts = apply_filters( 'ewd_otp_sales_rep_form_shortcode_atts', $sales_rep_atts );
// Extract the shortcode attributes
$args = shortcode_atts( $sales_rep_atts, $atts );
// Render booking form
ewd_otp_load_view_files();
// Possibly update order status and location from the front-end
$update = ewd_otp_possibly_update_order();
if ( $update ) {
$args['update_message'] = $update;
}
$sales_rep_form = new ewdotpViewSalesRepForm( $args );
$sales_rep_form->set_request_parameters();
$output = $sales_rep_form->render();
return $output;
}
Code file location:
order-tracking/order-tracking/includes/template-functions.php
Order Tracking [customer-order] Shortcode
The ‘customer-order’ shortcode is part of the Order Tracking Plugin. It generates a form for customers to submit their orders. The PHP code validates the customer’s order details, processes the order, and displays a success message or validation errors. If successful, it redirects the customer to a specified page.
Shortcode: [customer-order]
Parameters
Here is a list of all possible customer-order shortcode parameters and attributes:
location
– Specifies the location of an ordercustomer_name_field_text
– Changes the text of the customer name fieldcustomer_email_field_text
– Alters the text of the customer email fieldcustomer_notes_field_text
– Modifies the text of the customer notes fieldsuccess_redirect_page
– Redirects to a specific page after successful order submissionsubmit_text
– Changes the text of the submit button
Examples and Usage
Basic example – Implementing the shortcode without any additional attributes. This will use the default values set in the plugin settings.
[customer-order /]
Advanced examples
Using the shortcode to display a customer order form with a specific location. In this case, the form will only display orders related to the specified location.
[customer-order location="New York" /]
Customizing the text of the customer name, email, and notes fields. This allows you to personalize the form according to your needs.
[customer-order customer_name_field_text="Enter your full name" customer_email_field_text="Enter your email address" customer_notes_field_text="Any additional notes?" /]
Redirecting the customer to a specific page upon successful submission of the order form. This can be used to guide the customer to a thank you page or similar.
[customer-order success_redirect_page="https://yourwebsite.com/thank-you" /]
Changing the text of the submit button. This allows you to use a call to action that aligns with your brand language.
[customer-order submit_text="Place your order now!" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [customer-order]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'customer-order', 'ewd_otp_customer_order_form_shortcode' );
Shortcode PHP function:
function ewd_otp_customer_order_form_shortcode( $atts ) {
global $ewd_otp_controller;
if ( ! $ewd_otp_controller->permissions->check_permission( 'customer_orders' ) ) { return; }
// Define shortcode attributes
$customer_order_atts = array(
'location' => '',
'customer_name_field_text' => '',
'customer_email_field_text' => '',
'customer_notes_field_text' => '',
'success_redirect_page' => '',
'submit_text' => ''
);
// Create filter so addons can modify the accepted attributes
$customer_order_atts = apply_filters( 'ewd_otp_customer_order_form_shortcode_atts', $customer_order_atts );
// Extract the shortcode attributes
$args = shortcode_atts( $customer_order_atts, $atts );
// Handle order submission
if ( isset( $_POST['ewd_otp_form_type'] ) and $_POST['ewd_otp_form_type'] == 'customer_order_form' ) {
$args['order_submitted'] = true;
$order = new ewdotpOrder();
$status = $order->process_client_order_submission();
if ( ! $status ) {
$args['update_message'] = '';
foreach ( $order->validation_errors as $validation_error ) {
$args['update_message'] .= '<br />' . $validation_error['message'];
}
}
else {
$args['update_message'] = $ewd_otp_controller->settings->get_setting( 'label-customer-order-thank-you' ) . ' ' . $order->number;
if ( ! empty( $args['success_redirect_page'] ) ) { header( 'location:' . esc_url_raw( $args['success_redirect_page'] ) ); exit(); }
}
}
// Render booking form
ewd_otp_load_view_files();
$customer_order_form = new ewdotpViewCustomerOrderForm( $args );
$customer_order_form->set_request_parameters();
$output = $customer_order_form->render();
return $output;
}
Code file location:
order-tracking/order-tracking/includes/template-functions.php
Order Tracking [order-number-search] Shortcode
The ‘order-number-search’ shortcode is a feature of the Order Tracking Plugin. It allows users to search for their order using an order number. The shortcode creates a form on the webpage where users can input their order number. The form includes a text field for the order number and a submit button. The attributes of the form such as the tracking page URL, search label, search placeholder, and submit label can be customized according to the website’s requirements. The shortcode then returns the HTML output for the form, which is displayed on the webpage. The form submits the order number to the tracking page URL specified in the shortcode attributes.
Shortcode: [order-number-search]
Parameters
Here is a list of all possible order-number-search shortcode parameters and attributes:
tracking_page_url
– The URL of the page where tracking results are displayed.search_label
– The label displayed above the order number search field.search_placeholder
– The placeholder text within the order number search field.submit_label
– The text displayed on the tracking search button.
Examples and Usage
Basic example – This shortcode enables users to search for their order number through a simple input form.
[order-number-search /]
Advanced examples
Customizing the search form by setting a specific tracking page URL, customizing the search label, placeholder, and submit button label.
[order-number-search tracking_page_url="http://yourwebsite.com/tracking-page" search_label="Find your order:" search_placeholder="Your order number" submit_label="Search Now" /]
Using the shortcode to display a search form with a customized placeholder and submit button label. The form will display a text field with the placeholder “Enter your tracking number” and a submit button labeled “Track Order”.
[order-number-search search_placeholder="Enter your tracking number" submit_label="Track Order" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [order-number-search]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'order-number-search', 'ewd_otp_order_number_search_shortcode' );
Shortcode PHP function:
function ewd_otp_order_number_search_shortcode( $atts ) {
wp_enqueue_style( 'ewd-otp-css' );
$ewd_otp_order_number_search_atts = array(
'tracking_page_url' => '',
'search_label' => '',
'search_placeholder' => 'Please enter order number here',
'submit_label' => 'Track',
);
$args = shortcode_atts( $ewd_otp_order_number_search_atts, $atts );
$output = "<div class='ewd-otp-order-number-search'>";
$output .= "<form method='post' action='" . esc_attr( $args['tracking_page_url'] ) . "'>";
$output .= "<div class='ewd-otp-order-number-search-label'>" . sanitize_text_field( $args['search_label'] ) . "</div>";
$output .= "<div class='ewd-otp-order-number-search-input'><input type='text' name='tracking_number' placeholder='" . esc_attr( $args['search_placeholder'] ) . "'/></div>";
$output .= "<input type='submit' class='ewd-otp-order-number-search-submit' name='ewd_otp_order_number_search_submit' value='" . esc_attr( $args['submit_label'] ) . "' />";
$output .= "</form>";
$output .= "</div>";
return $output;
}
Code file location:
order-tracking/order-tracking/includes/template-functions.php
Conclusion
Now that you’ve learned how to embed the Order Tracking Plugin shortcodes, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply