Below, you’ll find a detailed guide on how to add the Post Content Shortcodes to your WordPress website, including their parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Post Content Shortcodes Plugin shortcodes not to show or not to work correctly.
Before starting, here is an overview of the Post Content Shortcodes Plugin and the shortcodes it provides:
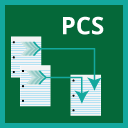
"Post Content Shortcodes is a versatile WordPress plugin that empowers users to insert different sections of content, including posts, pages, and user profiles, via simple shortcodes."
- [post-content]
- [post-list]
- [pcs-thumbnail]
- [pcs-post-url]
- [pcs-entry-classes]
Post Content [post-content] Shortcode
The ‘post-content’ shortcode retrieves and displays a specific post’s content. It accepts various attributes to customize the output, such as excluding current post, applying a content template, and stripping HTML tags. The code also handles post excerpts, post images, and post meta like author and date. It’s designed with flexibility and customization in mind, allowing for a variety of post display options.
Shortcode: [post-content]
Parameters
Here is a list of all possible post-content shortcode parameters and attributes:
id
– It identifies the specific post to display content from.blog_id
– It defines the blog from where the post will be retrieved.exclude_current
– It decides whether to exclude the current post or not.view_template
– It specifies the Content Template to render if Views is active.shortcodes
– It determines whether to keep or remove shortcodes in the post content and excerpt.strip_html
– It decides whether to strip HTML tags from the post content and excerpt.show_excerpt
– It controls the display of the post excerpt instead of the full content.excerpt_length
– It sets the maximum word count for the excerpt.show_image
– It controls the display of the post’s featured image.image_height
andimage_width
– They set the dimensions for the featured image.link_image
– It determines whether the featured image should be linked to the post.show_comments
– It controls the display of the post’s comments.show_date
andshow_author
– They control the display of the post’s date and author.show_title
– It controls the display of the post’s title.
Examples and Usage
Basic example – Displaying the content of a specific post using the post’s ID.
[post-content id=5 /]
Advanced examples
Displaying the content of a specific post from another blog in a multisite network. The blog ID is specified to retrieve the post.
[post-content id=5 blog_id=2 /]
Displaying the excerpt of a specific post instead of the full content. The ‘show_excerpt’ attribute is set to true.
[post-content id=5 show_excerpt=true /]
Displaying the content of a specific post but stripping all the HTML tags. The ‘strip_html’ attribute is set to true.
[post-content id=5 strip_html=true /]
Displaying the content of a specific post but without any shortcodes that might be present in the content. The ‘shortcodes’ attribute is set to false.
[post-content id=5 shortcodes=false /]
Displaying the content of a specific post along with the post’s featured image. The ‘show_image’ attribute is set to true.
[post-content id=5 show_image=true /]
Limiting the content of a specific post to a certain number of words. The ‘excerpt_length’ attribute is used to specify the number of words.
[post-content id=5 excerpt_length=50 /]
Displaying the content of a specific post along with the post’s comments. The ‘show_comments’ attribute is set to true.
[post-content id=5 show_comments=true /]
Displaying the content of a specific post along with the post’s title. The ‘show_title’ attribute is set to true.
[post-content id=5 show_title=true /]
Displaying the content of a specific post along with the post’s date and author. The ‘show_date’ and ‘show_author’ attributes are set to true.
[post-content id=5 show_date=true show_author=true /]
PHP Function Code
In case you have difficulties debugging what causing issues with [post-content]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'post-content', array( &$this, 'post_content' ) );
Shortcode PHP function:
function post_content( $atts=array() ) {
do_action( 'pcs_starting_post_content' );
global $wpdb;
$this->shortcode_atts = $this->_get_attributes( $atts );
extract( $this->shortcode_atts );
/**
* Attempt to avoid an endless loop
*/
if( ( is_array( $this->shortcode_atts ) && array_key_exists( 'exclude_current', $this->shortcode_atts ) && 'Do not exclude' !== $this->shortcode_atts['exclude_current'] ) && ( $id == $GLOBALS['post']->ID || empty( $id ) ) ) {
do_action( 'pcs_ending_post_content' );
return '';
}
/**
* Output a little debug info if necessary
*/
$this->debug( sprintf( 'Preparing to retrieve post content with the following args: %s', print_r( $this->shortcode_atts, true ) ) );
$p = $this->get_post_from_blog( $id, $blog_id );
if( empty( $p ) || is_wp_error( $p ) ) {
do_action( 'pcs_ending_post_content' );
return apply_filters( 'post-content-shortcodes-no-posts-error', __( '<p>No posts could be found that matched the specified criteria.</p>', 'post-content-shortcodes' ), $this->get_args( $this->shortcode_atts ) );
}
/**
* If Views is active, and the user has chosen to use a Content Template,
* render that instead of the default layout
*/
if ( function_exists( 'render_view_template' ) ) {
if ( array_key_exists( 'view_template', $this->shortcode_atts ) && ! empty( $this->shortcode_atts['view_template'] ) ) {
do_action( 'pcs_ending_post_content' );
return $this->do_view_template( $p );
}
}
$post_date = mysql2date( get_option( 'date_format' ), $p->post_date );
$post_author = get_userdata( $p->post_author );
if ( empty( $post_author ) || ! is_object( $post_author ) || ! isset( $post_author->display_name ) ) {
$post_author = (object) array( 'display_name' => '' );
$show_author = false;
}
if ( true !== $shortcodes ) {
$p->post_content = strip_shortcodes( $p->post_content );
$p->post_excerpt = strip_shortcodes( $p->post_excerpt );
}
if ( $strip_html ) {
if ( property_exists( $p, 'post_content' ) && ! empty( $p->post_content ) )
$p->post_content = strip_tags( apply_filters( 'the_content', $p->post_content, $p, $this->shortcode_atts ) );
if ( property_exists( $p, 'post_excerpt' ) && ! empty( $p->post_excerpt ) )
$p->post_excerpt = strip_tags( apply_filters( 'the_excerpt', $p->post_excerpt, $p, $this->shortcode_atts ) );
}
$content = $p->post_content;
if ( $show_excerpt ) {
$content = empty( $p->post_excerpt ) ? $p->post_content : $p->post_excerpt;
}
if ( intval( $excerpt_length ) && intval( $excerpt_length ) < str_word_count( $content ) ) {
$content = explode( ' ', $content );
$content = implode( ' ', array_slice( $content, 0, ( intval( $excerpt_length ) - 1 ) ) );
$content = force_balance_tags( $content );
$content .= apply_filters( 'post-content-shortcodes-read-more', ' <span class="read-more"><a href="' . get_permalink( $p->ID ) . '" title="' . apply_filters( 'the_title_attribute', $p->post_title, $p, $this->shortcode_atts ) . '">' . __( 'Read more', 'post-content-shortcodes' ) . '</a></span>', $p, $this->shortcode_atts );
}
if ( $show_image ) {
if ( empty( $image_height ) && empty( $image_width ) ) {
$image_size = apply_filters( 'post-content-shortcodes-default-image-size', 'thumbnail', $p, $this->shortcode_atts );
} else {
if ( empty( $image_height ) )
$image_height = 9999999;
if ( empty( $image_width ) )
$image_width = 9999999;
$image_size = array( intval( $image_width ), intval( $image_height ) );
}
if ( $link_image ) {
$link = get_permalink( $p->ID );
$p->post_thumbnail_linked = sprintf( '<a href="%s">%s</a>', $link, $p->post_thumbnail );
$content = apply_filters( 'post-content-shortcodes-include-thumbnail', $p->post_thumbnail_linked . $content, $p->post_thumbnail, $content, $p, $this->shortcode_atts );
} else {
$content = apply_filters( 'post-content-shortcodes-include-thumbnail', $p->post_thumbnail . $content, $p->post_thumbnail, $content, $p, $this->shortcode_atts );
}
}
if ( $show_comments ) {
$content .= $p->post_comments;
}
if ( $show_date && $show_author )
$content = apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted by <span class="post-author">%1$s</span> on <span class="post-date">%2$s</a>', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $this->shortcode_atts ) . $content;
elseif ( $show_date )
$content = apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted on %2$s', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $this->shortcode_atts ) . $content;
elseif ( $show_author )
$content = apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted by %s', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $this->shortcode_atts ) . $content;
if ( $show_title )
$content = apply_filters( 'post-content-shortcodes-title', '<h2>' . $p->post_title . '</h2>', $p->post_title, $p, $this->shortcode_atts ) . $content;
do_action( 'pcs_ending_post_content' );
return apply_filters( 'post-content-shortcodes-content', apply_filters( 'the_content', $content, $p, $this->shortcode_atts ), $p, $this->shortcode_atts );
}
Code file location:
post-content-shortcodes/post-content-shortcodes/classes/class-post-content-shortcodes.php
Post Content [post-list] Shortcode
The Post Content Shortcodes plugin’s shortcode is designed to create a list of posts. It allows customization of the post list based on various attributes like taxonomy, terms, post number, and more.
Shortcode: [post-list]
Parameters
Here is a list of all possible post-list shortcode parameters and attributes:
show_title
– Determines if the post title will be displayed or notnumberposts
– Sets the number of posts to be displayedtax_term
– Specifies the taxonomy terms to filter the poststax_name
– Specifies the taxonomy name to filter the postscategory
– Sets the category to filter the postsshow_excerpt
– Determines if the post excerpt will be displayed or notshow_image
– Determines if the post image will be displayed or notshow_author
– Determines if the post author will be displayed or notshow_date
– Determines if the post date will be displayed or notstrip_html
– Decides whether to strip HTML tags from the post contentexcerpt_length
– Sets the length of the post excerptread_more
– Determines if the ‘Read More’ link will be displayed or notshow_comments
– Determines if the post comments will be displayed or not
Examples and Usage
Basic example – Display a list of posts with their titles.
[post-list show_title=true /]
Advanced examples
Display a list of posts from a specific category, showing the title, author, and date of each post.
[post-list show_title=true show_author=true show_date=true tax_name='category' tax_term='news' /]
Display a list of posts with their titles and excerpts, limiting the number of posts to 5 and the excerpt length to 50 words.
[post-list show_title=true show_excerpt=true numberposts=5 excerpt_length=50 /]
Display a list of posts tagged ‘WordPress’, showing the title, image, and excerpt of each post, and linking the image to the post.
[post-list show_title=true show_image=true show_excerpt=true tax_name='tag' tax_term='WordPress' link_image=true /]
PHP Function Code
In case you have difficulties debugging what causing issues with [post-list]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'post-list', array( &$this, 'post_list' ) );
Shortcode PHP function:
function post_list( $atts=array() ) {
do_action( 'pcs_starting_post_list' );
if ( ! is_array( $atts ) )
$atts = array();
$args = $atts;
/**
* Set this shortcode to display the post title by default
*/
if ( ! array_key_exists( 'show_title', $atts ) )
$atts['show_title'] = true;
$atts = $this->_get_attributes( $atts );
$atts['posts_per_page'] = $atts['numberposts'];
$this->shortcode_atts = $atts;
$args = array_diff_key( $args, $atts );
$atts['tax_query'] = array();
if ( isset( $atts['tax_term'] ) && ! empty( $atts['tax_term'] ) && isset( $atts['tax_name'] ) && ! empty( $atts['tax_name'] ) ) {
$terms = explode( ' ', $atts['tax_term'] );
if ( count( $terms ) > 0 ) {
if ( 'tag' == $atts['tax_name'] ) {
if ( is_numeric( $terms[0] ) ) {
$atts['tag__in'] = $terms;
} else {
$atts['tag_slug__in'] = $terms;
}
} else if ( 'category' == $atts['tax_name'] ) {
if ( is_numeric( $terms[0] ) ) {
$atts['cat'] = implode( ',', $terms );
} else {
$atts['category_name'] = implode( ',', $terms );
}
} else {
if ( is_numeric( $terms[0] ) ) {
$field = 'id';
$terms = array_map( 'absint', $terms );
} else {
$field = 'slug';
}
$atts['tax_query'][] = array( 'taxonomy' => $atts['tax_name'], 'field' => $field, 'terms' => $terms );
}
}
}
$excluded_tax = apply_filters( 'post-content-shortcodes-excluded-taxonomies', array(
'csb_visibility',
'csb_clone',
) );
foreach ( $args as $k => $v ) {
if ( 'view_template' == $k || in_array( $k, $excluded_tax ) ) {
continue;
}
if ( is_numeric( $v ) )
$atts['tax_query'][] = array( 'taxonomy' => $k, 'field' => 'id', 'terms' => intval( $v ) );
else
$atts['tax_query'][] = array( 'taxonomy' => $k, 'field' => 'slug', 'terms' => $v );
}
if ( isset( $atts['category'] ) ) {
if ( is_numeric( $atts['category'] ) )
$atts['cat'] = $atts['category'];
else
$atts['category_name'] = $atts['category'];
}
/**
* Output a little debug info if necessary
*/
$this->debug( sprintf( 'Preparing to retrieve post list with the following args: %s', print_r( $atts, true ) ) );
$posts = $this->get_posts_from_blog( $atts, $atts['blog_id'] );
if( empty( $posts ) ) {
do_action( 'pcs_ending_post_list' );
return apply_filters( 'post-content-shortcodes-no-posts-error', __( '<p>No posts could be found that matched the specified criteria.</p>', 'post-content-shortcodes' ), $this->get_args( $atts ) );
}
/**
* If the user is using the WP Views plugin and specified a Content Template
* to use for the results, use that instead of the default layout
* @since 0.6
*/
if ( function_exists( 'render_view_template' ) ) {
if ( array_key_exists( 'view_template', $this->shortcode_atts ) && ! empty( $this->shortcode_atts['view_template'] ) ) {
$output = apply_filters( 'post-content-shortcodes-views-template-opening', '<div class="post-list">' );
add_filter( 'post_thumbnail_html', array( $this, 'do_post_thumbnail' ), 99 );
add_filter( 'post_link', array( $this, 'do_post_permalink' ), 99 );
$this->shortcode_atts['post_number'] = 1;
foreach ( $posts as $p ) {
$output .= $this->do_view_template( $p );
$this->shortcode_atts['post_number'] ++;
}
remove_filter( 'post_thumbnail_html', array( $this, 'do_post_thumbnail' ), 99 );
remove_filter( 'post_link', array( $this, 'do_post_permalink' ), 99 );
$output .= apply_filters( 'post-content-shortcodes-views-template-closing', '</div>' );
do_action( 'pcs_ending_post_list' );
return $output;
}
}
$output = apply_filters( 'post-content-shortcodes-open-list', '<ul class="post-list' . ( $atts['show_excerpt'] ? ' with-excerpt' : '' ) . ( $atts['show_image'] ? ' with-image' : '' ) . '">', $atts );
foreach( $posts as $p ) {
if ( ! is_object( $p ) )
continue;
if ( $atts['strip_html'] ) {
if ( property_exists( $p, 'post_content' ) && ! empty( $p->post_content ) )
$p->post_content = strip_tags( apply_filters( 'the_content', $p->post_content, $p, $atts ) );
if ( property_exists( $p, 'post_excerpt' ) && ! empty( $p->post_excerpt ) )
$p->post_excerpt = strip_tags( apply_filters( 'the_excerpt', $p->post_excerpt, $p, $atts ) );
}
$post_date = mysql2date( get_option( 'date_format' ), $p->post_date );
$post_author = get_userdata( $p->post_author );
$show_author = $atts['show_author'];
if ( empty( $post_author ) || ! is_object( $post_author ) || ! isset( $post_author->display_name ) ) {
$post_author = (object) array( 'display_name' => '' );
$show_author = false;
}
$li_classes = 'listed-post';
if ( $this->current_blog_id == $atts['blog_id'] && $this->current_post_id == $p->ID ) {
$li_classes .= ' current-post-item';
}
$output .= apply_filters( 'post-content-shortcodes-open-item', sprintf( '<li class="%s">', $li_classes ), $p->ID, $atts );
if ( $atts['show_title'] ) {
/**
* Applying filters to the link opening tag
* @uses apply_filters() to filter the title attribute
* @uses apply_filters() to filter the permalink
* @uses apply_filters() to filter the title attribute again?
* This portion of code probably applies filters too many times, but
* it is being left the way it is in order to preserve backward-compatibility
*/
$output .= apply_filters(
'post-content-shortcodes-item-link-open',
'<a class="pcs-post-title" href="' . $this->get_shortlink_from_blog( $p->ID, $atts['blog_id'] ) . '" title="' .
apply_filters(
'the_title_attribute',
$p->post_title
) .
'">',
apply_filters(
'the_permalink',
get_permalink( $p->ID )
),
apply_filters(
'the_title_attribute',
$p->post_title
),
$p,
$atts
);
$output .= apply_filters( 'the_title', $p->post_title, $p, $atts );
$output .= apply_filters( 'post-content-shortcodes-item-link-close', '</a>', $atts );
}
if ( $atts['show_author'] && $atts['show_date'] ) {
$output .= apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted by <span class="post-author">%1$s</span> on <span class="post-date">%2$s</a>', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $atts );
} elseif ( $atts['show_date'] ) {
$output .= apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted on <span class="post-date">%2$s</a>', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $atts );
} elseif ( $atts['show_author'] ) {
$output .= apply_filters( 'post-content-shortcodes-meta', '<p class="post-meta">' . sprintf( __( 'Posted by <span class="post-author">%1$s</span>', 'post-content-shortcodes' ), $post_author->display_name, $post_date ) . '</p>', $p, $atts );
}
if( $atts['show_excerpt'] ) {
$output .= '<div class="pcs-excerpt-wrapper">';
if ( stristr( $p->post_content, '<!--more-->' ) )
$p->post_content = force_balance_tags( substr( $p->post_content, 0, stripos( $p->post_content, '<!--more-->' ) ) );
}
if( $atts['show_image'] ) {
if ( $atts['link_image'] ) {
$output .= '<a href="' . $this->get_shortlink_from_blog( $p->ID, $atts['blog_id'] ) . '">' . $p->post_thumbnail . '</a>';
} else {
$output .= $p->post_thumbnail;
}
}
if( $atts['show_excerpt'] ) {
$excerpt = empty( $p->post_excerpt ) ? $p->post_content : $p->post_excerpt;
if( ! empty( $atts['excerpt_length'] ) && is_numeric( $atts['excerpt_length'] ) ) {
if ( ! $atts['shortcodes'] )
$excerpt = strip_shortcodes( $excerpt );
$excerpt = apply_filters( 'the_excerpt', $excerpt );
if( str_word_count( $excerpt ) > $atts['excerpt_length'] ) {
$excerpt = explode( ' ', $excerpt );
$excerpt = implode( ' ', array_slice( $excerpt, 0, ( $atts['excerpt_length'] - 1 ) ) );
$excerpt = force_balance_tags( $excerpt );
}
}
$read_more = $atts['read_more'] ?
apply_filters( 'post-content-shortcodes-read-more', ' <span class="read-more"><a href="' . $this->get_shortlink_from_blog( $p->ID, $atts['blog_id'] ) . '" title="' . apply_filters( 'the_title_attribute', $p->post_title ) . '">' . __( 'Read more', 'post-content-shortcodes' ) . '</a></span>', $p, $atts ) :
'';
$output .= '<div class="pcs-excerpt">' . apply_filters( 'post-content-shortcodes-list-excerpt', apply_filters( 'the_content', $excerpt . $read_more ), $p, $atts ) . '</div></div>';
}
if ( $atts['show_comments'] ) {
$output .= $p->post_comments;
}
$output .= apply_filters( 'post-content-shortcodes-close-item', '</li>', $atts );
}
$output .= apply_filters( 'post-content-shortcodes-close-list', '</ul>', $atts );
do_action( 'pcs_ending_post_list' );
return $output;
}
Code file location:
post-content-shortcodes/post-content-shortcodes/classes/class-post-content-shortcodes.php
Post Content [pcs-thumbnail] Shortcode
The Post Content Shortcodes plugin’s shortcode, ‘pcs-thumbnail’, is designed to display the post thumbnail. The function ‘do_post_thumbnail’ checks if the blog ID matches the shortcode attributes and if HTML content exists. If both conditions are met, it returns the HTML content. Otherwise, it returns the post thumbnail.
Shortcode: [pcs-thumbnail]
Examples and Usage
Basic example – Showcases how to use the ‘pcs-thumbnail’ shortcode to display the thumbnail of a blog post in a WordPress site.
[pcs-thumbnail blog_id=2 /]
Advanced examples
Illustrates the usage of the ‘pcs-thumbnail’ shortcode with multiple parameters. The shortcode will try to display the thumbnail of the specified blog post, but if it’s not found, it will display the thumbnail of the global post.
[pcs-thumbnail blog_id=2 html="
" /]
Another advanced example that demonstrates the use of the ‘pcs-thumbnail’ shortcode without specifying a blog ID. In this case, the shortcode will default to the global post’s thumbnail.
[pcs-thumbnail html="
" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [pcs-thumbnail]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'pcs-thumbnail', array( &$this, 'do_post_thumbnail' ) );
Shortcode PHP function:
function do_post_thumbnail( $html=null ) {
if ( $GLOBALS['blog_id'] == $this->shortcode_atts['blog_id'] && ! empty( $html ) )
return $html;
return $GLOBALS['post']->post_thumbnail;
}
Code file location:
post-content-shortcodes/post-content-shortcodes/classes/class-post-content-shortcodes.php
Post Content [pcs-post-url] Shortcode
The ‘pcs-post-url’ shortcode from the Post Content Shortcodes plugin retrieves the URL of a specific post. It compares the blog ID of the current blog to the blog ID specified in the shortcode. If they match and the link is not empty, it returns the link. Otherwise, it retrieves the short link from the specified blog using the post ID. This shortcode is useful for multi-site installations where you want to display links to specific posts across different blogs.
Shortcode: [pcs-post-url]
Examples and Usage
Basic example – A simple usage of the shortcode to get the URL of the current post.
[pcs-post-url blog_id=1 /]
Advanced examples
Use the shortcode to get the URL of the post from a different blog in a multisite network. Here, we are trying to get the URL of the post from blog with ID 2.
[pcs-post-url blog_id=2 /]
Here’s another advanced example where we use the shortcode to get the URL of a specific post. To do this, we pass the post ID as an attribute to the shortcode. In this case, we’re getting the URL for the post with ID 123 from blog with ID 2.
[pcs-post-url blog_id=2 post_id=123 /]
PHP Function Code
In case you have difficulties debugging what causing issues with [pcs-post-url]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'pcs-post-url', array( &$this, 'do_post_permalink' ) );
Shortcode PHP function:
function do_post_permalink( $link ) {
if ( $GLOBALS['blog_id'] == $this->shortcode_atts['blog_id'] && ! empty( $link ) )
return $link;
return $this->get_shortlink_from_blog( $GLOBALS['post']->ID, $this->shortcode_atts['blog_id'] );
}
Code file location:
post-content-shortcodes/post-content-shortcodes/classes/class-post-content-shortcodes.php
Post Content [pcs-entry-classes] Shortcode
The Post Content Shortcodes plugin’s shortcode ‘pcs-entry-classes’ is designed to manage CSS classes for entries. It accepts two attributes: ‘classes’ and ‘columns’. This shortcode allows users to specify CSS classes for individual posts. If the ‘columns’ attribute is set to a numeric value greater than 0, the plugin will calculate the column number for each post and assign additional CSS classes. This helps in creating responsive layouts.
Shortcode: [pcs-entry-classes]
Parameters
Here is a list of all possible pcs-entry-classes shortcode parameters and attributes:
classes
– Specifies additional classes for the entry.columns
– Defines the number of columns in the layout.
Examples and Usage
Basic example – A simple implementation of the shortcode without any specific classes or columns defined.
[pcs-entry-classes /]
Advanced examples
Defining specific classes for the entry. This example includes two classes ‘class1’ and ‘class2’.
[pcs-entry-classes classes="class1 class2" /]
Defining the number of columns. This example sets the number of columns to 3.
[pcs-entry-classes columns="3" /]
Combining both classes and columns. This example includes two classes ‘class1’ and ‘class2’ and sets the number of columns to 3.
[pcs-entry-classes classes="class1 class2" columns="3" /]
These examples demonstrate the flexibility of the ‘pcs-entry-classes’ shortcode. By adjusting the ‘classes’ and ‘columns’ parameters, you can customize the appearance and layout of your entries to suit your specific needs.
PHP Function Code
In case you have difficulties debugging what causing issues with [pcs-entry-classes]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'pcs-entry-classes', array( &$this, 'do_entry_classes' ) );
Shortcode PHP function:
function do_entry_classes( $atts=array() ) {
$atts = shortcode_atts( array( 'classes' => '', 'columns' => 0 ), $atts );
$classes = explode( ' ', $atts['classes'] );
if ( is_numeric( $atts['columns'] ) && $atts['columns'] > 0 ) {
$col = $this->shortcode_atts['post_number'] % $atts['columns'];
if ( 1 == $col ) {
$classes[] = 'first';
}
$classes[] = $col > 0 ? sprintf( 'column-%d', $col ) : sprintf( 'column-%d', $atts['columns'] );
}
return implode( ' ', $classes );
}
Code file location:
post-content-shortcodes/post-content-shortcodes/classes/class-post-content-shortcodes.php
Conclusion
Now that you’ve learned how to embed the Post Content Shortcodes Plugin shortcodes, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply