Below, you’ll find a detailed guide on how to add the Search & Filter Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Search & Filter Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the Search & Filter Plugin and the shortcodes it provides:
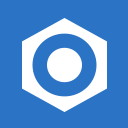
"Search & Filter is a simple yet powerful WordPress plugin that enhances your website's search functionality. It allows users to filter posts and pages to find relevant content quickly."
- [searchandfilter]
Search & Filter [searchandfilter] Shortcode
The Search and Filter shortcode is a powerful tool that enhances your website’s search functionality. It allows users to filter results based on various attributes like taxonomies, fields, and post types. This shortcode extracts attributes into variables and initializes them. It sets up default values for fields if none are provided. The shortcode then loops through these fields, setting up types and labels. It also configures the order of results and operator values. At the end, it returns a search filter form, enhancing the user’s search experience by providing more precise results. This makes the website more user-friendly and efficient.
Shortcode: [searchandfilter]
Parameters
Here is a list of all possible searchandfilter shortcode parameters and attributes:
fields
– Specifies the fields to include in the search.taxonomies
– Specifies the taxonomies to include in the search.submit_label
– The text displayed on the submit button.search_placeholder
– The placeholder text in the search field.types
– The type of fields to display (checkbox, radio, dropdown, etc).headings
– The headings for the fields in the search form.all_items_labels
– The labels for all items in the dropdowns.class
– The CSS class for the search form.post_types
– The type of posts to include in the search.hierarchical
– Whether to display hierarchical taxonomies.hide_empty
– Whether to hide empty taxonomies.order_by
– The parameter to order the search results by.show_count
– Whether to show the count of posts in each taxonomy.order_dir
– The direction to order the search results (asc/desc).operators
– The logical operators to use in the search query (and/or).add_search_param
– Whether to add the search parameter to the URL.empty_search_url
– The URL to redirect to if the search is empty.
Examples and Usage
Basic example – Display a search filter form with default settings.
[searchandfilter /]
Advanced examples
Customize the search filter form by specifying fields, submit label, and search placeholder.
[searchandfilter fields="title,category" submit_label="Find" search_placeholder="Enter keywords..."/]
Further customize the search filter form by specifying post types, order by, and order direction parameters.
[searchandfilter fields="title,category" submit_label="Find" search_placeholder="Enter keywords..." post_types="post,page" order_by="title" order_dir="asc"/]
Display a search filter form with multiple parameters including hierarchical view, hiding empty fields, showing count, and specifying the operator.
[searchandfilter fields="title,category" submit_label="Find" search_placeholder="Enter keywords..." post_types="post,page" hierarchical="1" hide_empty="1" show_count="1" operators="or"/]
PHP Function Code
In case you have difficulties debugging what causing issues with [searchandfilter]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'searchandfilter', array( $this, 'shortcode' ) );
Shortcode PHP function:
function shortcode( $atts, $content = null ) {
// Extract the attributes into variables.
extract(
shortcode_atts(
array(
'fields' => null,
'taxonomies' => null, // Will be deprecated - use `fields` instead.
'submit_label' => null,
'submitlabel' => null, // Will be deprecated - use `submit_label` instead.
'search_placeholder' => 'Search …',
'types' => '',
'type' => '', // Will be deprecated - use `types` instead.
'headings' => '',
'all_items_labels' => '',
'class' => '',
'post_types' => '',
'hierarchical' => '',
'hide_empty' => '',
'order_by' => '',
'show_count' => '',
'order_dir' => '',
'operators' => '',
'add_search_param' => '0',
'empty_search_url' => '',
),
$atts
)
);
// Init `fields`.
if ( $fields != null ) {
$fields = explode( ',', $fields );
} else {
$fields = explode( ',', $taxonomies );
}
$this->taxonomylist = $fields;
$nofields = count( $fields );
$add_search_param = (int) $add_search_param;
// Init `submitlabel`.
if ( $submitlabel !== null ) {
// Then the old "submitlabel" has been supplied.
if ( $submit_label === null ) {
// Then the new label has not been supplied so do nothing/
$submit_label = $submitlabel;
}
} elseif ( $submitlabel === null ) {
if ( $submit_label === null ) {
$submit_label = 'Submit';
}
}
// Init `post_types`.
if ( $post_types !== '' ) {
$post_types = explode( ',', $post_types );
} else {
if ( in_array( 'post_types', $fields, true ) ) {
$post_types = array( 'all' );
}
}
// Init `hierarchical`.
if ( $hierarchical != '' ) {
$hierarchical = explode( ',', $hierarchical );
} else {
$hierarchical = array( '' );
}
// Init `hide_empty`.
if ( $hide_empty != '' ) {
$hide_empty = explode( ',', $hide_empty );
} else {
$hide_empty = array( '' );
}
// Init `show_count`.
if ( $show_count !== '' ) {
$show_count = explode( ',', $show_count );
} else {
$show_count = array();
}
// Init `order_by`.
if ( $order_by !== '' ) {
$order_by = explode( ',', $order_by );
} else {
$order_by = array( '' );
}
// Init `order_dir`.
if ( $order_dir !== '' ) {
$order_dir = explode( ',', $order_dir );
} else {
$order_dir = array( '' );
}
// Init `operators`.
if ( $operators !== '' ) {
$operators = explode( ',', $operators );
} else {
$operators = array( '' );
}
// Init `labels`.
$labels = explode( ',', $headings );
if ( ! is_array( $labels ) ) {
$labels = array();
}
// Init `all_items_labels`.
$all_items_labels = explode( ',', $all_items_labels );
if ( ! is_array( $all_items_labels ) ) {
$all_items_labels = array();
}
// Init `types`.
if ( $types != null ) {
$types = explode( ',', $types );
} else {
$types = explode( ',', $type );
}
if ( ! is_array( $types ) ) {
$types = array();
}
// Loop through Fields and set up default vars.
for ( $i = 0; $i < $nofields; $i++ ) {
// Set up types.
if ( isset( $types[ $i ] ) ) {
// Check for post date field.
if ( $fields[ $i ] == 'post_date' ) {
if ( ( $types[ $i ] != 'date' ) && ( $types[ $i ] != 'daterange' ) ) {
// If not expected value use default.
$types[ $i ] = 'date';
}
} else {
// Everything else can use a standard form input - checkbox/radio/dropdown/list/multiselect.
if ( ( $types[ $i ] !== 'select' ) && ( $types[ $i ] !== 'checkbox' ) && ( $types[ $i ] !== 'radio' ) && ( $types[ $i ] !== 'list' ) && ( $types[ $i ] !== 'multiselect' ) ) {
$types[ $i ] = 'select'; // Use default.
}
}
} else {
// Omitted, so set default.
if ( $fields[ $i ] === 'post_date' ) {
$types[ $i ] = 'date';
} else {
$types[ $i ] = 'select';
}
}
// Setup labels.
if ( ! isset( $labels[ $i ] ) ) {
$labels[ $i ] = '';
}
// Setup all_items_labels.
if ( ! isset( $all_items_labels[ $i ] ) ) {
$all_items_labels[ $i ] = '';
}
if ( isset( $order_by[ $i ] ) ) {
if ( ( $order_by[ $i ] !== 'id' ) && ( $order_by[ $i ] !== 'name' ) && ( $order_by[ $i ] !== 'slug' ) && ( $order_by[ $i ] !== 'count' ) && ( $order_by[ $i ] !== 'term_group' ) ) {
$order_by[ $i ] = 'name'; // Use default - possible typo or use of unknown value.
}
} else {
$order_by[ $i ] = 'name'; // Use default.
}
if ( isset( $order_dir[ $i ] ) ) {
if ( ( $order_dir[ $i ] !== 'asc' ) && ( $order_dir[ $i ] !== 'desc' ) ) {
// Then order_dir is not a wanted value, set to default.
$order_dir[ $i ] = 'asc';
}
} else {
$order_dir[ $i ] = 'asc'; // Use default value.
}
if ( isset( $operators[ $i ] ) ) {
$operators[ $i ] = strtolower( $operators[ $i ] );
if ( ( $operators[ $i ] !== 'and' ) && ( $operators[ $i ] !== 'or' ) ) {
$operators[ $i ] = 'and'; // Else use default - possible typo or use of unknown value.
}
} else {
$operators[ $i ] = 'and'; // Use default value.
}
}
// Set all form defaults / dropdowns etc.
$this->set_defaults();
return $this->get_search_filter_form( $submit_label, $search_placeholder, $fields, $types, $labels, $hierarchical, $hide_empty, $show_count, $post_types, $order_by, $order_dir, $operators, $all_items_labels, $empty_search_url, $add_search_param, $class );
}
Code file location:
search-filter/search-filter/search-filter.php
Conclusion
Now that you’ve learned how to embed the Search & Filter Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply