Below, you’ll find a detailed guide on how to add the Simple Job Board Shortcodes to your WordPress website, including their parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Simple Job Board Plugin shortcodes not to show or not to work correctly.
Before starting, here is an overview of the Simple Job Board Plugin and the shortcodes it provides:
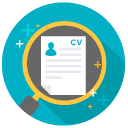
"Simple Job Board is a user-friendly WordPress plugin, designed to manage job listings on your website effortlessly. It's ideal for businesses seeking a streamlined recruitment process."
- [job_details]
- [jobpost]
Simple Job Board [job_details] Shortcode
The Simple Job Board shortcode ‘job_details’ is used to display job details on a webpage. It initiates several actions to enqueue scripts and display job content. This shortcode begins by enqueuing necessary scripts for the job board. It then triggers actions to start the single job content and listing. Within the job listing, it creates a ‘job-description’ div, fetching and displaying the content of the current post. After the content, it clears any floats, ending the job listing and content.
Shortcode: [job_details]
Examples and Usage
Basic example – Displays job details by referencing the job’s ID.
[job_details id=1 /]
PHP Function Code
In case you have difficulties debugging what causing issues with [job_details]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('job_details', array($this, 'sjb_job_form_function'));
Shortcode PHP function:
function sjb_job_form_function() {
do_action('sjb_enqueue_scripts');
do_action('sjb_single_job_content_start');
do_action('sjb_single_job_listing_start') ?>
<div class="job-description" id="job-desc">
<?php
global $post;
echo __( nl2br(get_post( $post->ID )->post_content) );
?>
</div>
<div class="clearfix"></div>
<?php
do_action('sjb_single_job_listing_end');
do_action('sjb_single_job_content_end');
}
Code file location:
simple-job-board/simple-job-board/includes/shortcodes/class-simple-job-board-shortcode-job-details.php
Simple Job Board [jobpost] Shortcode
The Simple Job Board shortcode allows users to display job listings on their WordPress site. This shortcode fetches and displays job posts based on specified parameters such as job type, location, and keywords. It supports pagination and allows users to search for jobs using filters. The shortcode also provides different layout options for job listings, including a grid or list view. It’s a versatile tool for any job board site.
Shortcode: [jobpost]
Parameters
Here is a list of all possible jobpost shortcode parameters and attributes:
posts
– Defines the number of job listings per pagecategory
– Filters job listings by specific categorytype
– Filters job listings by specific job typelocation
– Filters job listings by specific locationkeywords
– Filters job listings by specific keywordsorder
– Sets the order of job listings (ASC/DESC)search
– Enables or disables the search functionalitylayout
– Sets the layout of job listings (grid or list)
Examples and Usage
Basic example – Displaying a job board with a set number of posts
[jobpost posts=10]
With this shortcode, you’re telling WordPress to display a job board with a maximum of 10 job posts. This is a simple way to control the number of job listings that appear on a page.
Advanced examples
Displaying a job board with job listings from a specific category
[jobpost category="Marketing" posts=5]
In this example, the shortcode is used to display a job board with a maximum of 5 job posts, but only those that are categorized under ‘Marketing’. This is useful when you want to create a job board page that is specific to a certain job category.
Displaying a job board with job listings based on location and job type
[jobpost location="New York" type="Full Time"]
This shortcode displays a job board that lists only full-time jobs located in New York. It’s a great way to customize your job board based on specific job criteria.
Displaying a job board with job listings based on keywords
[jobpost keywords="Web Developer" posts=10]
In this example, the shortcode is used to display a job board with a maximum of 10 job posts that match the keyword ‘Web Developer’. This can be handy when you want to create a job board page specific to a certain job role or skill.
PHP Function Code
In case you have difficulties debugging what causing issues with [jobpost]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('jobpost', array($this, 'jobpost_shortcode'));
Shortcode PHP function:
function jobpost_shortcode($atts, $content) {
/**
* Enqueue Frontend Scripts.
*
* @since 2.2.4
*/
do_action('sjb_enqueue_scripts');
ob_start();
global $job_query;
// Shortcode Default Array
$shortcode_args = array(
'posts' => apply_filters('sjb_posts_per_page', 15),
'category' => '',
'type' => '',
'location' => '',
'keywords' => '',
'order' => 'DESC',
'search' => 'true',
'layout' => '',
);
$atts = is_array($atts) ? apply_filters('sjb_shortcode_atts', array_map('sanitize_text_field', $atts)) : '';
// Combine User Defined Shortcode Attributes with Known Attributes
$shortcode_args = shortcode_atts(apply_filters('sjb_output_jobs_defaults', $shortcode_args, $atts), $atts);
// Get paged variable.
if (get_query_var('paged')) {
$paged = (int) get_query_var('paged');
} elseif (get_query_var('page')) {
$paged = (int) get_query_var('page');
} else {
$paged = 1;
}
// WP Query Default Arguments
$args = apply_filters(
'sjb_output_jobs_args', array(
'post_status' => 'publish',
'posts_per_page' => sanitize_text_field($shortcode_args['posts']),
'post_type' => 'jobpost',
'paged' => $paged,
'order' => sanitize_text_field($shortcode_args['order']),
'jobpost_category' => (!empty($_GET['selected_category']) && -1 != $_GET['selected_category'] ) ? sanitize_text_field($_GET['selected_category']) : sanitize_text_field($shortcode_args['category']),
'jobpost_job_type' => (!empty($_GET['selected_jobtype']) && -1 != $_GET['selected_jobtype'] ) ? sanitize_text_field($_GET['selected_jobtype']) : sanitize_text_field($shortcode_args['type']),
'jobpost_location' => (!empty($_GET['selected_location']) && -1 != $_GET['selected_location'] ) ? sanitize_text_field($_GET['selected_location']) : sanitize_text_field($shortcode_args['location']),
's' => ( NULL != filter_input(INPUT_GET, 'search_keywords') ) ? sanitize_text_field($_GET['search_keywords']) : '',
), $atts
);
// Job Query
$job_query = new WP_Query($args);
/**
* Fires before listing jobs on job listing page.
*
* @since 2.2.0
*/
do_action('sjb_job_filters_before');
/**
* Template -> Job Listing Wrapper Start:
*
* - SJB Starting of Job Listing Wrapper
*/
get_simple_job_board_template('listing/listing-wrapper-start.php');
if ('false' != strtolower($shortcode_args['search']) && !empty($shortcode_args['search'])):
/**
* Template -> Job Filters:
*
* - Keywords Search.
* - Job Category Filter.
* - Job Type Filter.
* - Job Location Filter.
*
* Search jobs by keywords, category, location & type.
*/
get_simple_job_board_template('job-filters.php', array('per_page' => $shortcode_args['posts'], 'order' => $shortcode_args['order'], 'categories' => $shortcode_args['category'], 'job_types' => $shortcode_args['type'], 'atts' => $atts, 'location' => $shortcode_args['location'], 'keywords' => $shortcode_args['keywords']));
endif;
/**
* Template -> Job Listing Start:
*
* - SJB Starting of Job List
*/
get_simple_job_board_template('listing/job-listings-start.php');
/**
* Fires before listing jobs on job listing page.
*
* @since 2.2.0
*/
do_action('sjb_job_listing_before');
if ($job_query->have_posts()):
global $counter, $post_count;
$counter = 1;
$post_count = $job_query->post_count;
while ($job_query->have_posts()): $job_query->the_post();
//Backward Compatibility
if ($shortcode_args['layout']) {
// Display the user defined job listing view
if ('grid' === $shortcode_args['layout']) {
get_simple_job_board_template('content-job-listing-grid-view.php' );
} elseif ('list' === $shortcode_args['layout']) {
get_simple_job_board_template('content-job-listing-list-view.php');
}
} elseif (FALSE !== get_option('job_board_listing_view')) {
$job_board_listing_view = get_option('job_board_listing_view');
// Display the user defined job listing view
if ('grid-view' === $job_board_listing_view) {
get_simple_job_board_template('content-job-listing-grid-view.php');
} elseif ('list-view' === $job_board_listing_view) {
get_simple_job_board_template('content-job-listing-list-view.php');
}
} else {
get_simple_job_board_template('content-job-listing-list-view.php');
}
endwhile;
/**
* Template -> Pagination:
*
* - Add Pagination to Resulted Jobs.
*/
get_simple_job_board_template('listing/job-pagination.php', array('job_query' => $job_query));
else:
/**
* Template -> No Job Found:
*
* - Display Message on No Job Found.
*/
get_simple_job_board_template_part('listing/content-no-jobs-found');
endif;
wp_reset_postdata();
/**
* Fires after listing jobs on job listing page.
*
* @since 2.2.0
*/
do_action('sjb_job_listing_after');
/**
* Template -> Job Listing End:
*
* - SJB Ending of Job List.
*/
get_simple_job_board_template('listing/job-listings-end.php');
/**
* Template -> Job Listing Wrapper End:
*
* - SJB Endding of Job Listing Wrapper
*/
get_simple_job_board_template('listing/listing-wrapper-end.php');
$html = ob_get_clean();
/**
* Filter -> Modify the Job Listing Shortcode
*
* @since 2.2.0
*
* @param HTML $html Job Listing HTML Structure.
*/
return apply_filters('sjb_job_listing_shortcode', $html . do_shortcode($content), $atts);
}
Code file location:
simple-job-board/simple-job-board/includes/shortcodes/class-simple-job-board-shortcode-jobpost.php
Conclusion
Now that you’ve learned how to embed the Simple Job Board Plugin shortcodes, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply