Below, you’ll find a detailed guide on how to add the Spacer Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Spacer Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the Spacer Plugin and the shortcodes it provides:
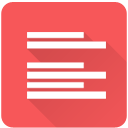
"Spacer is a WordPress plugin that allows users to add precise spacing between elements on their website. It provides an easy and efficient way to manage visual appeal and layout organization."
- [spacer]
Spacer [spacer] Shortcode
The Spacer shortcode is a useful tool in WordPress that allows for the creation of space between elements on a page. This shortcode takes various parameters such as height, mobile height, class, ID, and style. It determines the height of the spacer based on whether the device is mobile or desktop. If a mobile height is set, it uses that; otherwise, it defaults to the desktop height. The shortcode also allows for custom background images, colors, and CSS styles. It returns a span element with the specified class, style, and height, effectively creating a spacer in your content.
Shortcode: [spacer]
Parameters
Here is a list of all possible spacer shortcode parameters and attributes:
height
– Sets the desktop height of the spacermheight
– Sets the mobile height of the spacerclass
– Adds a specific CSS class to the spacerid
– Defines a unique identifier for the spacerstyle
– Allows for inline CSS styling of the spacer
Examples and Usage
Basic example – Displaying a spacer with a specific height
[spacer height="20" /]
Adjusting the height of the spacer to 20px. If no unit is specified, the shortcode defaults to pixels.
Advanced examples
Creating a spacer with a specific height for mobile devices, and a different height for desktop devices.
[spacer height="30" mheight="20" /]
In this example, the spacer will be 30px high on desktop devices and 20px high on mobile devices. This is useful for creating responsive designs that look good on all devices.
Adding a custom CSS class to the spacer
[spacer height="30" class="my-custom-class" /]
This example shows how to add a custom CSS class to the spacer. This can be useful if you want to apply additional styles to the spacer using CSS.
Using the shortcode to create a spacer with a specific ID and style
[spacer id="mySpacer" style="background-color: red;" /]
In this example, the spacer has an ID of “mySpacer” and a style attribute that sets its background color to red. This allows you to target the spacer with JavaScript or CSS for further customization.
PHP Function Code
In case you have difficulties debugging what causing issues with [spacer]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('spacer', array($this, 'addShortcodeHandler'));
Shortcode PHP function:
function addShortcodeHandler($atts, $content = null) {
extract(shortcode_atts(array( "height" => '', "mheight" => '', "class" => '', "id" => '', "style" => '' ), $atts));
$activespacer = $this->activespacer($id);
//prep variables
$spacer_css = "";
$classes = "";
//prep mobile height, if it's empty we will use desktop height. if set to 0 we will hide the spacer on mobile devices.
$mobile_height = "";
$mobile_height_inline = "";
$mobile_height_default = $activespacer["mobile_height_default"];
//first check for inline height, then check default mobile height
if(isset($mheight) && $mheight != ""){
$mobile_height = $mheight;
$mobile_height_inline = $mheight;
}elseif(isset($mobile_height_default) && $mobile_height_default != ""){
$mobile_height = $activespacer["mobile_height"];
$mobile_height_default = $mobile_height;
}
//determine the height to use for the spacer. if it's a mobile device and there is a mobile height set, use that. otherwise use desktop height
if( function_exists('wp_is_mobile') && wp_is_mobile() && (isset($mobile_height) && $mobile_height != "")) {
$mobileunit = $activespacer["mobileunit"];
if(isset($mobile_height_inline) && $mobile_height_inline != "") {
if ($mobile_height_inline > 0 ) {
$spacer_css .= "padding-top: " . $mobile_height_inline . ";";
} elseif($mobile_height_inline < 0) {
$spacer_css .= "margin-top: " . $mobile_height_inline . ";";
} elseif($mobile_height_inline == 0){
$spacer_css .= "display:none;";
}
} elseif(isset($mobile_height_default) && $mobile_height_default != ""){
if($mobile_height_default > 0){
$spacer_css .= "padding-top: " . $mobile_height_default . $mobileunit.";";
}elseif($mobile_height_default < 0){
$spacer_css .= "margin-top: " . $mobile_height_default . $mobileunit. ";";
}elseif($mobile_height_default == 0){;
$spacer_css .= "display:none;";
}
}
} elseif($height=="default"){ //there is no mobile height set. use the desktop default height
//for now assume positive. in a sec add logic for if negative
$checkheight = $activespacer["checkheight"];
$checkunit = $activespacer["checkunit"];
if($checkheight > 0){
$spacer_css .= "padding-top: " . $checkheight . $checkunit.";";
}elseif($checkheight < 0){
$spacer_css .= "margin-top: " . $checkheight . $checkunit. ";";
}
} elseif ($height > 0 ) { #no default for desktop, use positive inline height
$spacer_css .= "padding-top: " . $height . ";";
} elseif($height < 0) { #no positive inline for desktop, use negative inline height
$spacer_css .= "margin-top: " . $height . ";";
}
//custom background image
/* $bg = $activespacer["bg"];
if(!empty($bg)) {
$spacer_css .= "background: url(".$bg.");";
}*/
//custom background image position
//$spacer_css .= $this->background_position();
//background color
/* $bgcolor = $activespacer["bgcolor"];
if(!empty($bgcolor)) {
$spacer_css .= "background-color:".$bgcolor.";";
}*/
//classes
$defaultclasses = $activespacer["defaultclasses"];
$classes .= $defaultclasses;
if(!empty($class)){
$classes .= " ".$class;
}
if(has_filter('spacer_add_css')) {
$spacer_css = apply_filters('spacer_add_css', $spacer_css, $activespacer);
}
//styles
$defaultstyle = $activespacer["defaultstyle"];
$spacer_css .= $defaultstyle;
if(!empty($style)){
$spacer_css .= " ".$style;
}
$classes = esc_attr($classes);
$spacer_css = esc_attr($spacer_css);
//create the spacer after all settings have been loaded
return '<span class="'.$classes.'" style="display:block;clear:both;height: 0px;'.$spacer_css.'"></span>';
}
Code file location:
spacer/spacer/index.php
Conclusion
Now that you’ve learned how to embed the Spacer Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply