Below, you’ll find a detailed guide on how to add the Easy Appointments Shortcodes to your WordPress website, including their parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the Easy Appointments Plugin shortcodes not to show or not to work correctly.
Before starting, here is an overview of the Easy Appointments Plugin and the shortcodes it provides:
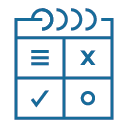
"Easy Appointments is a user-friendly WordPress plugin that simplifies your scheduling process. It allows for easy booking, managing, and tracking of appointments, enhancing efficiency and organization."
- [ea_standard]
- [ea_bootstrap]
- [ea_full_calendar]
Easy Appointments [ea_standard] Shortcode
The Easy Appointments shortcode enables the creation of a booking form with customizable settings. It provides options like defining the start of the week, default date, min/max date, and more.
Shortcode: [ea_standard]
Parameters
Here is a list of all possible ea_standard shortcode parameters and attributes:
scroll_off
– disables automatic scrolling to the appointment form.save_form_content
– saves the content of the form for later use.start_of_week
– sets the starting day of the week.default_date
– sets the default date for the appointment.min_date
– sets the minimum date that can be selected for an appointment.max_date
– sets the maximum date that can be selected for an appointment.show_remaining_slots
– displays the remaining slots for an appointment.show_week
– displays the week of the selected date.
Examples and Usage
Basic example – Displaying the standard appointment form with default settings
[ea_standard /]
Advanced examples
Displaying the standard appointment form with no scrolling, saving form content enabled, and a default date set to a specific date.
[ea_standard scroll_off=true save_form_content=true default_date='2022-12-31' /]
Displaying the standard appointment form with a specific start of the week (0 for Sunday, 1 for Monday, etc.), a minimum and maximum date for appointments, and showing remaining slots.
[ea_standard start_of_week=1 min_date='2022-01-01' max_date='2022-12-31' show_remaining_slots='1' /]
Displaying the standard appointment form with a specific start of the week (0 for Sunday, 1 for Monday, etc.), a minimum and maximum date for appointments, and not showing the week in the calendar.
[ea_standard start_of_week=1 min_date='2022-01-01' max_date='2022-12-31' show_week='0' /]
PHP Function Code
In case you have difficulties debugging what causing issues with [ea_standard]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('ea_standard', array($this, 'standard_app'));
Shortcode PHP function:
function standard_app($atts)
{
$code_params = shortcode_atts(array(
'scroll_off' => false,
'save_form_content' => true,
'start_of_week' => get_option('start_of_week', 0),
'default_date' => date('Y-m-d'),
'min_date' => null,
'max_date' => null,
'show_remaining_slots' => '0',
'show_week' => '0',
), $atts);
// all those values are used inside JS code part, escape all values to be JS strings
foreach ($code_params as $key => $value) {
if ($value === null || $value === '0' || $value === '1' || strlen($value) < 4) {
continue;
}
// also remove '{', '}' brackets because no settings needs that
$code_params[$key] = esc_js(str_replace(array('{','}',';'), array('','',''), $value));
}
$settings = $this->options->get_options();
// unset secret
unset($settings['captcha.secret-key']);
$settings['check'] = wp_create_nonce('ea-bootstrap-form');
$settings['scroll_off'] = $code_params['scroll_off'];
$settings['start_of_week'] = $code_params['start_of_week'];
$settings['default_date'] = $code_params['default_date'];
$settings['min_date'] = $code_params['min_date'];
$settings['max_date'] = $code_params['max_date'];
$settings['show_remaining_slots'] = $code_params['show_remaining_slots'];
$settings['save_form_content'] = $code_params['save_form_content'];
$settings['show_week'] = $code_params['show_week'];
$settings['trans.please-select-new-date'] = __('Please select another day', 'easy-appointments');
$settings['trans.date-time'] = __('Date & time', 'easy-appointments');
$settings['trans.price'] = __('Price', 'easy-appointments');
// datetime format
$settings['time_format'] = $this->datetime->convert_to_moment_format(get_option('time_format', 'H:i'));
$settings['date_format'] = $this->datetime->convert_to_moment_format(get_option('date_format', 'F j, Y'));
$settings['default_datetime_format'] = $this->datetime->convert_to_moment_format($this->datetime->default_format());
$settings['trans.nonce-expired'] = __('Form validation code expired. Please refresh page in order to continue.', 'easy-appointments');
$settings['trans.internal-error'] = __('Internal error. Please try again later.', 'easy-appointments');
$settings['trans.ajax-call-not-available'] = __('Unable to make ajax request. Please try again later.', 'easy-appointments');
$customCss = $settings['custom.css'];
$customCss = strip_tags($customCss);
$customCss = str_replace(array('<?php', '?>', "\t"), array('', '', ''), $customCss);
$meta = $this->models->get_all_rows("ea_meta_fields", array(), array('position' => 'ASC'));
wp_enqueue_script('underscore');
wp_enqueue_script('ea-validator');
wp_enqueue_script('ea-front-end');
if (empty($settings['css.off'])) {
wp_enqueue_style('ea-jqueryui-style');
wp_enqueue_style('ea-frontend-style');
wp_enqueue_style('ea-admin-awesome-css');
}
if (!empty($settings['captcha.site-key'])) {
wp_enqueue_script('ea-google-recaptcha');
}
$custom_form = $this->generate_custom_fields($meta);
// add custom CSS
ob_start();
$this->output_inline_ea_settings($settings, $customCss);
// GET TEMPLATE
require $this->utils->get_template_path('booking.overview.tpl.php');
?>
<script type="text/javascript">
var ea_ajaxurl = "<?php echo admin_url('admin-ajax.php'); ?>";
</script>
<div class="ea-standard">
<form>
<div class="step">
<div class="block"></div>
<label class="ea-label"><?php echo esc_html(__($this->options->get_option_value("trans.location"), 'easy-appointments')) ?></label><select
name="location" data-c="location"
class="filter"><?php $this->get_options("locations") ?></select>
</div>
<div class="step">
<div class="block"></div>
<label class="ea-label"><?php echo esc_html(__($this->options->get_option_value("trans.service"), 'easy-appointments')) ?></label><select
name="service" data-c="service" class="filter"
data-currency="<?php echo $this->options->get_option_value("trans.currency") ?>"><?php $this->get_options("services") ?></select>
</div>
<div class="step">
<div class="block"></div>
<label class="ea-label"><?php echo esc_html(__($this->options->get_option_value("trans.worker"), 'easy-appointments')) ?></label><select
name="worker" data-c="worker" class="filter"><?php $this->get_options("staff") ?></select>
</div>
<div class="step calendar" class="filter">
<div class="block"></div>
<div class="date"></div>
</div>
<div class="step" class="filter">
<div class="block"></div>
<div class="time"></div>
</div>
<div class="step final">
<div class="block"></div>
<p class="section"><?php _e('Personal information', 'easy-appointments'); ?></p>
<small><?php _e('Fields with * are required', 'easy-appointments'); ?></small>
<br>
<?php echo $custom_form; ?>
<br>
<p class="section"><?php _e('Booking overview', 'easy-appointments'); ?></p>
<div id="booking-overview"></div>
<?php if (!empty($settings['show.iagree'])) : ?>
<p>
<label
style="font-size: 65%; width: 80%;" class="i-agree"><?php _e('I agree with terms and conditions', 'easy-appointments'); ?>
* : </label><input style="width: 15%;" type="checkbox" name="iagree"
data-rule-required="true"
data-msg-required="<?php _e('You must agree with terms and conditions', 'easy-appointments'); ?>">
</p>
<br>
<?php endif; ?>
<?php if (!empty($settings['gdpr.on'])) : ?>
<p>
<label
style="font-size: 65%; width: 80%;" class="gdpr"><?php echo esc_html($settings['gdpr.label']);?>
* : </label><input style="width: 15%;" type="checkbox" name="iagree"
data-rule-required="true"
data-msg-required="<?php echo esc_attr($settings['gdpr.message']);?>">
</p>
<br>
<?php endif; ?>
<?php if (!empty($settings['captcha.site-key'])) : ?>
<div style="width: 100%" class="g-recaptcha" data-sitekey="<?php echo esc_attr($settings['captcha.site-key']);?>"></div><br>
<?php endif; ?>
<div style="display: inline-flex;">
<?php echo apply_filters('ea_checkout_button', '<button class="ea-btn ea-submit">' . __('Submit', 'easy-appointments') . '</button>'); ?>
<button class="ea-btn ea-cancel"><?php _e('Cancel', 'easy-appointments'); ?></button>
</div>
</div>
</form>
<div id="ea-loader"></div>
</div>
<?php
apply_filters('ea_checkout_script', '');
$content = ob_get_clean();
// compress output
if ($this->options->get_option_value('shortcode.compress', '1') === '1') {
$content = preg_replace('/\s+/', ' ', $content);
}
return $content;
}
Code file location:
easy-appointments/easy-appointments/src/frontend.php
Easy Appointments [ea_bootstrap] Shortcode
The Easy Appointments shortcode is used to create a customizable booking form. It allows you to define parameters like location, service, and worker. It also enables you to set the form’s width, layout, and date range. Additionally, it allows you to control the display of remaining slots and blocked days. This shortcode also supports localization for a better user experience.
Shortcode: [ea_bootstrap]
Parameters
Here is a list of all possible ea_bootstrap shortcode parameters and attributes:
location
– Specifies the ID of the location for the appointment.service
– Specifies the ID of the service for the appointment.worker
– Specifies the ID of the worker for the appointment.width
– Adjusts the width of the form, default is 400px.scroll_off
– If set to true, disables the scroll feature.save_form_content
– If true, saves the content of the form.layout_cols
– Specifies the number of columns in the layout, default is 1.start_of_week
– Sets the start day of the week, default is 0 (Sunday).rtl
– If set to ‘1’, switches layout to right-to-left.default_date
– Sets the default date for the appointment.min_date
– Sets the minimum selectable date for the appointment.max_date
– Sets the maximum selectable date for the appointment.show_remaining_slots
– If ‘1’, displays the remaining slots for appointments.show_week
– If ‘1’, displays the week numbers.cal_auto_select
– If ‘1’, enables automatic date selection in the calendar.block_days
– Blocks specific days from being selected.block_days_tooltip
– Sets the tooltip text for blocked days.select_placeholder
– Sets the placeholder text for select fields.
Examples and Usage
Basic example – Display a booking form with default settings.
[ea_bootstrap /]
Advanced examples
Display a booking form with a specified width and default date.
[ea_bootstrap width="500px" default_date="2022-12-31" /]
Display a booking form with a specified location, service, and worker.
[ea_bootstrap location="1" service="2" worker="3" /]
Display a booking form with specified minimum and maximum dates for booking.
[ea_bootstrap min_date="2022-01-01" max_date="2022-12-31" /]
Display a booking form with a specific layout and start of week.
[ea_bootstrap layout_cols="2" start_of_week="1" /]
Display a booking form with a specific language direction (RTL).
[ea_bootstrap rtl="1" /]
Display a booking form with a specific placeholder for selection fields.
[ea_bootstrap select_placeholder="Please select" /]
Display a booking form with a specified blocked days and a tooltip for those days.
[ea_bootstrap block_days="1,2,3" block_days_tooltip="These days are blocked" /]
Display a booking form with the form content saving option turned off.
[ea_bootstrap save_form_content="false" /]
Display a booking form with the option to show remaining slots and the week number.
[ea_bootstrap show_remaining_slots="1" show_week="1" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [ea_bootstrap]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('ea_bootstrap', array($this, 'ea_bootstrap'));
Shortcode PHP function:
function ea_bootstrap($atts)
{
$code_params = shortcode_atts(array(
'location' => null,
'service' => null,
'worker' => null,
'width' => '400px',
'scroll_off' => false,
'save_form_content' => true,
'layout_cols' => '1',
'start_of_week' => get_option('start_of_week', 0),
'rtl' => '0',
'default_date' => date('Y-m-d'),
'min_date' => null,
'max_date' => null,
'show_remaining_slots' => '0',
'show_week' => '0',
'cal_auto_select' => '1',
'block_days' => null,
'block_days_tooltip' => '',
'select_placeholder' => '-'
), $atts);
// check params
apply_filters('ea_bootstrap_shortcode_params', $atts);
// all those values are used inside JS code part, escape all values to be JS strings
foreach ($code_params as $key => $value) {
if ($value === null || $value === '0' || $value === '1' || strlen($value) < 4) {
continue;
}
// also remove '{', '}' brackets because no settings needs that
$code_params[$key] = esc_js(str_replace(array('{','}',';'), array('','',''), $value));
}
// used inside template ea_bootstrap.tpl.php
$location_id = $code_params['location'];
$service_id = $code_params['service'];
$worker_id = $code_params['worker'];
$settings = $this->options->get_options();
// unset secret
unset($settings['captcha.secret-key']);
$settings['check'] = wp_create_nonce('ea-bootstrap-form');
$settings['width'] = $code_params['width'];
$settings['scroll_off'] = $code_params['scroll_off'];
$settings['layout_cols'] = $code_params['layout_cols'];
$settings['start_of_week'] = $code_params['start_of_week'];
$settings['rtl'] = $code_params['rtl'];
$settings['default_date'] = $code_params['default_date'];
$settings['min_date'] = $code_params['min_date'];
$settings['max_date'] = $code_params['max_date'];
$settings['show_remaining_slots'] = $code_params['show_remaining_slots'];
$settings['show_week'] = $code_params['show_week'];
$settings['save_form_content'] = $code_params['save_form_content'];
$settings['cal_auto_select'] = $code_params['cal_auto_select'];
$settings['block_days'] = $code_params['block_days'] !== null ? explode(',', $code_params['block_days']) : null;
$settings['block_days_tooltip'] = $code_params['block_days_tooltip'];
// LOCALIZATION
$settings['trans.please-select-new-date'] = __('Please select another day', 'easy-appointments');
$settings['trans.personal-informations'] = __('Personal information', 'easy-appointments');
$settings['trans.field-required'] = __('This field is required.', 'easy-appointments');
$settings['trans.error-email'] = __('Please enter a valid email address', 'easy-appointments');
$settings['trans.error-name'] = __('Please enter at least 3 characters.', 'easy-appointments');
$settings['trans.error-phone'] = __('Please enter at least 3 digits.', 'easy-appointments');
$settings['trans.fields'] = __('Fields with * are required', 'easy-appointments');
$settings['trans.email'] = __('Email', 'easy-appointments');
$settings['trans.name'] = __('Name', 'easy-appointments');
$settings['trans.phone'] = __('Phone', 'easy-appointments');
$settings['trans.comment'] = __('Comment', 'easy-appointments');
$settings['trans.overview-message'] = __('Please check your appointment details below and confirm:', 'easy-appointments');
$settings['trans.booking-overview'] = __('Booking overview', 'easy-appointments');
$settings['trans.date-time'] = __('Date & time', 'easy-appointments');
$settings['trans.submit'] = __('Submit', 'easy-appointments');
$settings['trans.cancel'] = __('Cancel', 'easy-appointments');
$settings['trans.price'] = __('Price', 'easy-appointments');
$settings['trans.iagree'] = __('I agree with terms and conditions', 'easy-appointments');
$settings['trans.field-iagree'] = __('You must agree with terms and conditions', 'easy-appointments');
$settings['trans.slot-not-selectable'] = __('You can\'t select this time slot!\'', 'easy-appointments');
$settings['trans.nonce-expired'] = __('Form validation code expired. Please refresh page in order to continue.', 'easy-appointments');
$settings['trans.internal-error'] = __('Internal error. Please try again later.', 'easy-appointments');
$settings['trans.ajax-call-not-available'] = __('Unable to make ajax request. Please try again later.', 'easy-appointments');
// datetime format
$settings['time_format'] = $this->datetime->convert_to_moment_format(get_option('time_format', 'H:i'));
$settings['date_format'] = $this->datetime->convert_to_moment_format(get_option('date_format', 'F j, Y'));
$settings['default_datetime_format'] = $this->datetime->convert_to_moment_format($this->datetime->default_format());
// CUSTOM CSS
$customCss = $settings['custom.css'];
$customCss = strip_tags($customCss);
$customCss = str_replace(array('<?php', '?>', "\t"), array('', '', ''), $customCss);
unset($settings['custom.css']);
if ($settings['form.label.above'] === '1') {
$settings['form_class'] = 'ea-form-v2';
}
$rows = $this->models->get_all_rows("ea_meta_fields", array(), array('position' => 'ASC'));
$rows = apply_filters( 'ea_form_rows', $rows);
$settings['MetaFields'] = $rows;
wp_enqueue_script('underscore');
wp_enqueue_script('ea-validator');
wp_enqueue_script('ea-bootstrap');
wp_enqueue_script('ea-front-bootstrap');
if (empty($settings['css.off'])) {
wp_enqueue_style('ea-bootstrap');
wp_enqueue_style('ea-admin-awesome-css');
wp_enqueue_style('ea-frontend-bootstrap');
}
if (!empty($settings['captcha.site-key'])) {
wp_enqueue_script('ea-google-recaptcha');
}
if (!empty($settings['captcha3.site-key'])) {
wp_enqueue_script('ea-google-recaptcha-v3', "https://www.google.com/recaptcha/api.js?render={$settings['captcha3.site-key']}");
}
ob_start();
$this->output_inline_ea_settings($settings, $customCss);
// FORM TEMPLATE
if ($settings['rtl'] == '1') {
require EA_SRC_DIR . 'templates/ea_bootstrap_rtl.tpl.php';
} else {
require EA_SRC_DIR . 'templates/ea_bootstrap.tpl.php';
}
// OVERVIEW TEMPLATE
require $this->utils->get_template_path('booking.overview.tpl.php');
?>
<div class="ea-bootstrap bootstrap"></div><?php
// load scripts if there are some
apply_filters('ea_checkout_script', '');
$content = ob_get_clean();
// compress output
if ($this->options->get_option_value('shortcode.compress', '1') === '1') {
$content = preg_replace('/\s+/', ' ', $content);
}
return $content;
}
Code file location:
easy-appointments/easy-appointments/src/frontend.php
Easy Appointments [ea_full_calendar] Shortcode
The Easy Appointments shortcode ‘ea_full_calendar’ is designed to display a full calendar on your WordPress site. It includes customizable parameters such as ‘location’, ‘service’, ‘worker’, and ‘color’. It also allows for setting the start of the week, date format, and more.
Shortcode: [ea_full_calendar]
Parameters
Here is a list of all possible ea_full_calendar shortcode parameters and attributes:
location
– Specifies the location for the appointments.service
– Specifies the service for the appointments.worker
– Specifies the worker for the appointments.color
– Enables color-coding of services if set to ‘true’.start_of_week
– Sets the starting day of the week.rtl
– Enables right-to-left text direction if set to ‘1’.default_date
– Sets the default date on the calendar.min_date
– Sets the minimum selectable date on the calendar.max_date
– Sets the maximum selectable date on the calendar.time_format
– Defines the format for displaying times.display_event_end
– Displays event end time if set to ‘1’.hide_cancelled
– Hides cancelled appointments if set to ‘1’.show_remaining_slots
– Shows remaining slots if set to ‘1’.show_week
– Shows week numbers if set to ‘1’.title_field
– Specifies the field to use for event titles.default_view
– Sets the default view of the calendar (e.g., ‘month’, ‘week’, ‘day’).views
– Sets the available views on the calendar (e.g., ‘month’, ‘week’, ‘day’).day_names_short
– Specifies the short names for days of the week.day_names
– Specifies the full names for days of the week.month_names_short
– Specifies the short names for months.month_names
– Specifies the full names for months.button_labels
– Specifies the labels for calendar buttons.month_header_format
– Sets the format for month headers.week_header_format
– Sets the format for week headers.day_header_format
– Sets the format for day headers.column_header_format
– Sets the format for column headers.
Examples and Usage
Basic example – Display a full calendar with default settings
[ea_full_calendar /]
Advanced example – Display a full calendar with a specified location, service, and worker. The calendar will also start on a specified date and use a 24-hour time format.
[ea_full_calendar location="1" service="2" worker="3" default_date="2022-01-01" time_format="H:i" /]
More Advanced example – Display a full calendar with a specified location, service, and worker. The calendar will also start on a specified date, use a 24-hour time format, and only display appointments for the current month. The calendar will also be displayed in the ‘week’ view by default.
[ea_full_calendar location="1" service="2" worker="3" default_date="2022-01-01" min_date="2022-01-01" max_date="2022-01-31" time_format="H:i" default_view="week" /]
Customized example – Display a full calendar with custom day and month names. The calendar will also display the end time of events and hide cancelled appointments.
[ea_full_calendar day_names_short="Sun,Mon,Tue,Wed,Thu,Fri,Sat" day_names="Sunday,Monday,Tuesday,Wednesday,Thursday,Friday,Saturday" month_names_short="Jan,Feb,Mar,Apr,May,Jun,Jul,Aug,Sep,Oct,Nov,Dec" month_names="January,February,March,April,May,June,July,August,September,October,November,December" display_event_end="1" hide_cancelled="1" /]
PHP Function Code
In case you have difficulties debugging what causing issues with [ea_full_calendar]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode('ea_full_calendar', array($this, 'ea_full_calendar'));
Shortcode PHP function:
function ea_full_calendar($atts)
{
$code_params = shortcode_atts(array(
'location' => null,
'service' => null,
'worker' => null,
'color' => false,
'start_of_week' => get_option('start_of_week', 0),
'rtl' => '0',
'default_date' => date('Y-m-d'),
'min_date' => null,
'max_date' => null,
'time_format' => 'h(:mm)t',
'display_event_end' => '0',
'hide_cancelled' => '0',
'show_remaining_slots' => '0',
'show_week' => '0',
'title_field' => 'name',
'default_view' => 'month', // basicWeek, basicDay, agendaDay, agendaWeek
'views' => 'month,basicWeek,basicDay',
'day_names_short' => 'Sun,Mon,Tue,Wed,Thu,Fri,Sat',
'day_names' => 'Sunday,Monday,Tuesday,Wednesday,Thursday,Friday,Saturday',
'month_names_short' => 'Jan,Feb,Mar,Apr,May,Jun,Jul,Aug,Sep,Oct,Nov,Dec',
'month_names' => 'January,February,March,April,May,June,July,August,September,October,November,December',
'button_labels' => 'today,month,week,day,list',
'month_header_format' => 'MMM YYYY',
'week_header_format' => 'MMM DD, YYYY',
'day_header_format' => 'MMM DD, YYYY',
'column_header_format' => null,
), $atts);
// all those values are used inside JS code part, escape all values to be JS strings
foreach ($code_params as $key => $value) {
if ($value === null || $value === '0' || $value === '1' || strlen($value) < 4) {
continue;
}
// also remove '{', '}' brackets because no settings needs that
$code_params[$key] = esc_js(str_replace(array('{','}',';'), array('','',''), $value));
}
// scripts that are going to be used
wp_enqueue_script('underscore');
wp_enqueue_script('ea-validator');
wp_enqueue_script('ea-full-calendar');
// add thickbox styles
wp_enqueue_style('thickbox.css', includes_url('/js/thickbox/thickbox.css'), null, '1.0');
wp_enqueue_style('ea-full-calendar-style');
wp_enqueue_style('ea-full-calendar-custom-css');
$customCss = $this->options->get_option_value('custom.css', '');
$id = uniqid();
$show_week_numbers = $code_params['show_week'] === '1' ? 'true' : 'false';
$is_rtl = $code_params['rtl'] === '1' ? 'true' : 'false';
/**
* Convert string: 'Sun,Mon,Tue,Wed,Thu,Fri,Sat' to string
* "['Sun','Mon','Tue','Wed','Thu','Fri','Sat']" etc
*/
$day_names_short = $this->convert_csv_to_js_array_of_strings($code_params['day_names_short']);
$day_names = $this->convert_csv_to_js_array_of_strings($code_params['day_names']);
$month_names_short = $this->convert_csv_to_js_array_of_strings($code_params['month_names_short']);
$month_names = $this->convert_csv_to_js_array_of_strings($code_params['month_names']);
$button_labels = explode(',', $code_params['button_labels']);
// set it as optional
$location_param = $code_params['location'] !== null ? "location: '{$code_params['location']}'," : '';
$service_param = $code_params['service'] !== null ? "service: '{$code_params['service']}'," : '';
$worker_param = $code_params['worker'] !== null ? "worker: '{$code_params['worker']}'," : '';
$service_color = $code_params['color'] === 'service' ? "color: true," : '';
$display_end_time = $code_params['display_event_end'] ? 'true' : 'false';
$event_click_link = '';
// event link
if (!empty($this->options->get_option_value('fullcalendar.event.show'))) {
$event_click_link = <<<EOT
element.addClass('thickbox');
element.addClass('ea-full-calendar-dialog-event');
element.attr('href', wpApiSettings.root + 'easy-appointments/v1/appointment/' + event.id + '?hash=' + event.hash + '&_wpnonce=' + wpApiSettings.nonce + '&width=100%&height=100%');
element.attr('title', '#' + event.id + ' - ' + _.escape(event.title));
EOT;
}
$column_header_format = '';
if ($code_params['column_header_format'] !== null) {
$column_header_format = "columnHeaderFormat: '{$code_params['column_header_format']}',";
}
$script_section = <<<EOT
<style>{$customCss}</style>
<script>
jQuery(document).ready(function() {
jQuery('#ea-calendar-color-map-{$id}').find('.status').hover(
function(event) {
var el = jQuery(event.target);
var classSelector = '.' + el.data('class');
jQuery('#ea-full-calendar-{$id}').find('.fc-event:not(' + classSelector + ')').animate({ opacity: 1/2 }, 200);
},
function(event){
jQuery('#ea-full-calendar-{$id}').find('.fc-event').animate({ opacity: 1 }, 100);
});
jQuery('#ea-full-calendar-{$id}').fullCalendar({
header: {
left: 'prev,next today',
center: 'title',
right: '{$code_params['views']}'
},
dayNamesShort: {$day_names_short},
dayNames: {$day_names},
monthNamesShort: {$month_names_short},
monthNames: {$month_names},
buttonText: {
today: '{$button_labels[0]}',
month: '{$button_labels[1]}',
week: '{$button_labels[2]}',
day: '{$button_labels[3]}',
list: '{$button_labels[4]}'
},
views: {
month: {
titleFormat: '{$code_params['month_header_format']}',
},
week: {
titleFormat: '{$code_params['week_header_format']}',
},
day: {
titleFormat: '{$code_params['day_header_format']}',
}
},
isRTL: {$is_rtl},
defaultView: '{$code_params['default_view']}',
showNonCurrentDates: false,
timeFormat: '{$code_params['time_format']}',
{$column_header_format}
displayEventEnd: {$display_end_time},
weekNumbers: {$show_week_numbers},
firstDay: {$code_params['start_of_week']},
defaultDate: '{$code_params['default_date']}',
navLinks: true, // can click day/week names to navigate views
editable: false,
eventLimit: true, // allow "more" link when too many events
events: {
url: wpApiSettings.root + 'easy-appointments/v1/appointments',
type: 'GET',
data: {
_wpnonce: wpApiSettings.nonce,
hide_cancelled: {$code_params['hide_cancelled']},
{$location_param}
{$service_param}
{$worker_param}
{$service_color}
title_field: '{$code_params['title_field']}',
},
error: function() {
alert('there was an error while fetching events!');
},
textColor: 'white' // a non-ajax option
},
eventClick: function(calEvent, jsEvent, view) {
// console.log(calEvent, jsEvent, view);
},
eventRender: function(event, element) {
var statusMapping = {
canceled: 'graffit',
confirmed: 'darkgreen',
pending: 'grape',
reserved: 'darkblue'
}
element.addClass(statusMapping[event.status]);
{$event_click_link}
}
});
});
</script>
EOT;
// wp_add_inline_script( 'ea-full-calendar', $script_section);
$statuses = $this->logic->getStatus();
$status_label = __('Status', 'easy-appointments');
$status_html = <<<EOT
<div class="fc">
<div id="ea-calendar-color-map-{$id}" class="ea-calendar-color-map fc-view-container">
<div>{$status_label}</div>
<div data-class="grape" class="fc-event status grape">{$statuses['pending']}</div>
<div data-class="darkgreen" class="fc-event status darkgreen">{$statuses['confirmed']}</div>
<div data-class="darkblue" class="fc-event status darkblue">{$statuses['reservation']}</div>
<div data-class="graffit" class="fc-event status graffit">{$statuses['canceled']}</div>
</div>
</div>
EOT;
// html and status legend
$html = <<<EOT
<div id="ea-full-calendar-{$id}"></div>
EOT;
if (!$service_color) {
$html .= $status_html;
}
$html .= $script_section;
return $html;
}
Code file location:
easy-appointments/easy-appointments/src/shortcodes/fullcalendar.php
Conclusion
Now that you’ve learned how to embed the Easy Appointments Plugin shortcodes, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply