Below, you’ll find a detailed guide on how to add the SEO Slider Shortcode to your WordPress website, including its parameters, examples, and PHP function code. Additionally, we’ll assist you with common issues that might cause the SEO Slider Plugin shortcode not to show or not to work correctly.
Before starting, here is an overview of the SEO Slider Plugin and the shortcodes it provides:
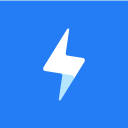
"SEO Slider is a WordPress plugin designed to enhance your website's SEO through interactive sliders. It simplifies the process of creating, customizing, and managing sliders, boosting your site's visibility."
- [seo_slider_shortcode]
SEO Slider [seo_slider_shortcode] Shortcode
The SEO Slider shortcode allows users to create and customize a slider with various options. It fetches data such as dots, arrows, loop, autoplay, effect, duration, transition, mobile, desktop, overlay, text, and slides from the post meta, and displays them in a slick slider.
Shortcode: [seo_slider_shortcode]
Parameters
Here is a list of all possible seo_slider_shortcode shortcode parameters and attributes:
id
– Unique identifier of the sliderdots
– Displays navigation dots on the sliderarrows
– Shows navigation arrows on the sliderloop
– Enables or disables slider repetitionautoplay
– Automatically starts the slider on page loadeffect
– Determines the transition effect of the sliderduration
– Sets the time between each slide transitiontransition
– Defines the speed of slide transitionmobile
– Sets slider height for mobile devicesdesktop
– Sets slider height for desktop displaysoverlay
– Changes the background color of the slider overlaytext
– Alters the color of the text in the sliderslides
– Contains the information for each individual slide
Examples and Usage
Basic example – Displaying a slider with default settings by referencing its ID.
[slider id=1 /]
Advanced examples
Displaying a slider with custom settings. In this example, the slider will have arrows and dots navigation, loop enabled, autoplay enabled with duration of 2000ms, fade effect, transition speed of 500ms, and specific heights for mobile and desktop views.
[slider id=1 arrows=true dots=true loop=true autoplay=true duration=2000 effect=true transition=500 mobile=500 desktop=700 /]
Displaying a slider with custom text and overlay color. In this example, the text color is set to white (#ffffff) and the overlay color is set to black with 50% opacity (#80000000).
[slider id=1 text=#ffffff overlay=#80000000 /]
It is important to note that the values for the attributes in the shortcode must be set in the post meta of the slider post. If not, the default values will be used.
PHP Function Code
In case you have difficulties debugging what causing issues with [seo_slider_shortcode]
shortcode, check below the related PHP functions code.
Shortcode line:
add_shortcode( 'slider', 'seo_slider_shortcode' );
Shortcode PHP function:
function seo_slider_shortcode( $atts ) {
$atts = shortcode_atts(
[
'id' => 1,
],
$atts
);
$output = '';
$id = (int) $atts['id'];
$prefix = 'seo_slider_';
$breakpoint = apply_filters( 'seo_slider_breakpoint', 640 );
$dots = esc_html( get_post_meta( $id, $prefix . 'dots', true ) ? 'true' : 'false' );
$arrows = esc_html( get_post_meta( $id, $prefix . 'arrows', true ) ? 'true' : 'false' );
$loop = esc_html( get_post_meta( $id, $prefix . 'loop', true ) ? 'true' : 'false' );
$autoplay = esc_html( get_post_meta( $id, $prefix . 'autoplay', true ) ? 'true' : 'false' );
$effect = esc_html( get_post_meta( $id, $prefix . 'effect', true ) === 'true' ? 'true' : 'false' );
$duration = esc_html( get_post_meta( $id, $prefix . 'duration', true ) );
$transition = esc_html( get_post_meta( $id, $prefix . 'transition', true ) );
$mobile = esc_html( get_post_meta( $id, $prefix . 'mobile', true ) );
$desktop = esc_html( get_post_meta( $id, $prefix . 'desktop', true ) );
$overlay = esc_html( get_post_meta( $id, $prefix . 'overlay', true ) );
$text = esc_html( get_post_meta( $id, $prefix . 'text', true ) );
$slides = get_post_meta( $id, $prefix . 'slides', true );
$js = "
jQuery( document ).ready( function($) {
$( '.slick-slider-$id' ).slick( {
dots: $dots,
infinite: $loop,
speed: $transition,
arrows: $arrows,
autoplay: $autoplay,
autoplaySpeed: $duration,
fade: $effect,
slidesToShow: 1 ,
slidesToScroll: 1,
lazyLoad: 'ondemand',
mobileFirst: true
} );
} );
";
$css = "
.slick-slider-$id {
height: ${mobile}px;
}
.slick-slider-$id,
.slick-slider-$id p,
.slick-slider-$id h1,
.slick-slider-$id h2,
.slick-slider-$id h3,
.slick-slider-$id h4,
.slick-slider-$id h5,
.slick-slider-$id h6,
.slick-slider-$id li {
color: $text;
}
.slick-slider-$id .slick-overlay {
background-color: $overlay;
}
@media (min-width: ${breakpoint}px) {
.slick-slider-$id {
height: ${desktop}px;
}
}
";
if ( apply_filters( 'seo_slider_output_inline_js', true ) ) {
$output .= sprintf( '<script>%s</script>', $js );
} else {
wp_add_inline_script( seo_slider_get_slug(), $js );
}
if ( apply_filters( 'seo_slider_output_inline_css', false ) ) {
$output .= sprintf( '<style>%s</style>', seo_slider_minify_css( $css ) );
} else {
wp_add_inline_style( seo_slider_get_slug(), seo_slider_minify_css( $css ) );
}
ob_start();
do_action( 'seo_slider_before_slider', $id );
?>
<section class="slick-slider slick-slider-<?php echo esc_attr( $id ); ?>"
role="banner" itemscope itemtype="http://schema.org/ImageGallery">
<?php $slide_id = 1; ?>
<?php foreach ( $slides as $slide ) : ?>
<?php do_action( 'seo_slider_before_slide', $slide ); ?>
<figure
class="slick-slide slick-slide-<?php esc_attr_e( $slide_id++ ); ?>"
itemprop="associatedMedia" itemscope
itemtype="http://schema.org/ImageObject">
<?php
$img_id = $slide['seo_slider_image_id'] ?? false;
$img_size = apply_filters( 'seo_slider_image_size', 'slider' );
$img_html = wp_get_attachment_image( $img_id, $img_size, false, [
'class' => 'slick-image',
'itemprop' => 'image',
] );
?>
<?php if ( isset( $slide['seo_slider_image_id'] ) ) :
echo apply_filters( 'seo_slider_image_output', $img_html, $img_id, $img_size );
endif; ?>
<div class="slick-overlay"></div>
<?php do_action( 'seo_slider_before_wrap', $slide ); ?>
<div class="slick-wrap">
<?php do_action( 'seo_slider_before_content', $slide ); ?>
<div class="slick-content" itemprop="description">
<?php if ( isset( $slide['seo_slider_content'] ) ) :
printf( apply_filters(
'seo_slider_content_output',
do_shortcode( wp_kses_post( wpautop( $slide['seo_slider_content'] ) ) ),
$slide['seo_slider_content']
) );
endif; ?>
</div>
<?php do_action( 'seo_slider_after_content', $slide ); ?>
</div>
<?php do_action( 'seo_slider_after_wrap', $slide ); ?>
</figure>
<?php do_action( 'seo_slider_after_slide', $slide ); ?>
<?php endforeach; ?>
</section>
<?php
do_action( 'seo_slider_after_slider', $id );
$output .= ob_get_clean();
return apply_filters( 'seo_slider_output', $output );
}
Code file location:
seo-slider/seo-slider/includes/shortcode.php
Conclusion
Now that you’ve learned how to embed the SEO Slider Plugin shortcode, understood the parameters, and seen code examples, it’s easy to use and debug any issue that might cause it to ‘not work’. If you still have difficulties with it, don’t hesitate to leave a comment below.
Leave a Reply